void replaceChar(char* str, char chr, char* new_str) { int str_len = strlen(new_str); int len = strlen(str); for (int i = 0; i < len; i++) { if (str[i] == chr) { str = realloc(str, len + str_len); memmove(str + i + strlen(new_str), str + i + 1, len - i - 1); memcpy(str + i, new_str, strlen(new_str)); len += strlen(new_str) - 1; } } } int main() { char *buf = strdup("hello123456"); printf("buf=%s\n", buf); replaceChar(buf, 'o', "aaaaaaaaaaaaaa"); printf("buf=%s\n", buf); free(buf); return 0; }为什么这段代码会异常
时间: 2024-02-14 22:22:31 浏览: 140
这段代码会异常的原因是 realloc 函数可能会改变指针 str 所指向的内存地址,导致原来的指针变成了野指针,从而引发访问非法内存的错误。在循环中,每次调用 realloc 都可能改变 str 的指向,因此在使用 str 时就可能出现问题。此外,还有可能出现内存泄漏的问题,因为每次调用 realloc 都会分配新的内存,但是之前分配的内存并没有被释放。另外,这段代码还存在一个潜在的问题,就是在使用 memcpy 函数时并没有考虑新字符串和旧字符串的长度关系,可能会出现内存越界的错误。
相关问题
replaceChar
replaceChar是一个用于替换字符串中指定字符的函数。它接受三个参数:原始字符串、要替换的字符和替换后的字符。函数会遍历原始字符串,将所有与要替换的字符相同的字符替换为指定的替换字符,并返回替换后的字符串。
以下是replaceChar函数的示例代码:
```python
def replaceChar(string, char_to_replace, replacement_char):
new_string = ""
for char in string:
if char == char_to_replace:
new_string += replacement_char
else:
new_string += char
return new_string
```
使用replaceChar函数可以方便地替换字符串中的字符。例如,如果我们有一个字符串"Hello, World!",想要将其中的逗号替换为感叹号,可以这样调用replaceChar函数:
```python
original_string = "Hello, World!"
char_to_replace = ","
replacement_char = "!"
new_string = replaceChar(original_string, char_to_replace, replacement_char)
print(new_string) # 输出:Hello! World!
```
主函数如何写charvalue; //构造函数//拷贝构造函数//析构函数 //赋值函数,如setValue(charnewvalue) //返回有效字符的个数//返回某个位置的字符//替换某个位置的字符//选做:合并两个字符串,如 Concat(MyString&) //主函数如何写
,charsecondString); //选做:在字符串中查找某个字符或子串的位置,如 find(charsub) 或 find(MyStringsubString);
class MyString{
private:
char* str;
int size;
public:
MyString(){
str = new char[1];
str[0] = '\0';
size = 0;
}
MyString(const char* s){
size = strlen(s);
str = new char[size+1];
for(int i=0; i<=size; i++)
str[i] = s[i];
}
MyString(const MyString& other){
size = other.size;
str = new char[size+1];
for(int i=0; i<=size; i++)
str[i] = other.str[i];
}
~MyString(){
delete[] str;
}
MyString& operator=(const MyString& other){
if(this == &other)
return *this;
delete[] str;
size = other.size;
str = new char[size+1];
for(int i=0; i<=size; i++)
str[i] = other.str[i];
return *this;
}
void setValue(char newvalue){
str[0] = newvalue;
size = 1;
}
int getValidSize() const{
return size;
}
char getChar(int pos) const{
if(pos >= size)
return '\0';
return str[pos];
}
void replaceChar(int pos, char c){
if(pos >= size)
return;
str[pos] = c;
}
MyString Concat(const MyString& other) const{
MyString result;
result.size = size + other.size;
result.str = new char[result.size+1];
int i=0;
for(; i<size; i++)
result.str[i] = str[i];
for(; i<result.size; i++)
result.str[i] = other.str[i-size];
return result;
}
int find(const char* sub) const{
int sub_size = strlen(sub);
for(int i=0; i<=size-sub_size; i++){
int j=0;
while(j<sub_size && str[i+j]==sub[j])
j++;
if(j==sub_size)
return i;
}
return -1;
}
int find(const MyString& sub) const{
return find(sub.str);
}
};
阅读全文
相关推荐











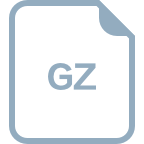