python的划线怎么画箭头
时间: 2023-02-10 10:45:30 浏览: 242
可以使用 matplotlib 库来画箭头。首先,需要导入 matplotlib 库和使用 pyplot 模块中的 arrow 函数。
例如,下面的代码可以画一条从 (0,0) 到 (1,1) 的线,并在线的末端放置一个箭头:
```
import matplotlib.pyplot as plt
plt.arrow(0, 0, 1, 1, head_width=0.1, head_length=0.2, fc='k', ec='k')
plt.show()
```
该代码会显示一个图形窗口,其中包含一条从 (0,0) 到 (1,1) 的线,并在线的末端放置了一个箭头。
arrow 函数的参数列表如下:
- x, y:线的起点坐标
- dx, dy:线的终点坐标
- head_width:箭头的宽度
- head_length:箭头的长度
- fc, ec:箭头的前景色和边缘色
可以根据需要调整这些参数来调整箭头的外观。
相关问题
python 画坐标轴加箭头
在Python中,通常使用matplotlib库来绘制图表,包括添加坐标轴和箭头。下面是一个简单的例子,展示如何创建一个带有坐标轴和箭头的直角坐标系:
```python
import matplotlib.pyplot as plt
from mpl_toolkits.axes_grid1 import host_subplot
# 创建一个新的图形
fig = plt.figure()
ax = host_subplot(111) # 主坐标轴
# 设置坐标轴范围
ax.set_xlim(-5, 5)
ax.set_ylim(-5, 5)
# 添加网格线
ax.grid(True)
# 添加刻度标记和标签
ax.xaxis.set_major_locator(plt.MultipleLocator(1))
ax.yaxis.set_major_locator(plt.MultipleLocator(1))
ax.set_xlabel('X轴')
ax.set_ylabel('Y轴')
# 添加箭头示例 (这里只演示了x轴正方向的箭头,其他方向可以类似操作)
arrowprops = dict(arrowstyle="->", connectionstyle="angle,angleA=0,angleB=-90")
ax.annotate("", xy=(5, 0), xytext=(-5, 0), arrowprops=arrowprops)
# 显示图形
plt.show()
```
在这个例子中,`annotate()`函数用于添加箭头,它需要两个点作为起始和结束位置,并提供了一些属性来定制箭头样式。
python如何画出增长的箭头,
在Python中,你可以使用matplotlib库来创建动态的图形,包括带有增长箭头的图表。箭头通常用于表示数据变化的方向。这里是一个简单的例子,展示如何结合`pyplot`模块和`FuncAnimation`函数来创建这样的图表:
```python
import matplotlib.pyplot as plt
from matplotlib.animation import FuncAnimation
def draw_growth(x, y):
ax = plt.gca()
line, = ax.plot(x, y) # 创建线
arrowprops = dict(arrowstyle='->', connectionstyle="angle,angleA=0,angleB=90") # 定义箭头属性
artist = ax.annotate("", xy=(x[-1], y[-1]), xytext=(x[0], y[0]), arrowprops=arrowprops) # 绘制箭头
return line, artist
# 假设你有数据点list_data = [(0, 0), (1, 10), (2, 20), ...]
fig, ax = plt.subplots()
line, artist = draw_growth(list_data[::len(list_data)//5]) # 每隔一段时间更新一次箭头位置
def update(frame):
line.set_data(*zip(*list_data[:frame]))
artist.xy = list_data[frame] # 更新箭头的位置
return line,
ani = FuncAnimation(fig, update, frames=len(list_data), interval=100, repeat=True) # 设置动画帧数和间隔时间
plt.show()
阅读全文
相关推荐
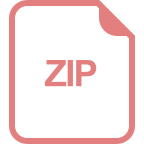
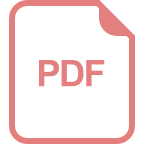
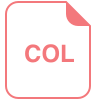











