03.设计完整程序,由自定义数据类型struct Student{unsigned ID;string Name;int Score;//……}的数组 stu[]={{1004,"AAAA",90},{1002,"BBBB" ,80},{1005,"CCCC",85},{1007," DDDD",75} ,{1001 , ''EEE'',65} }生成集合 set 或 multiset 或 unordered_set 等并输出(要求以学号ID为 Key)。
时间: 2024-03-01 21:55:40 浏览: 25
以下是生成集合并输出的C++代码实现:
```c++
#include <iostream>
#include <set>
#include <unordered_set>
using namespace std;
struct Student {
unsigned ID;
string Name;
int Score;
};
bool operator<(const Student& s1, const Student& s2) {
return s1.ID < s2.ID;
}
int main() {
Student stu[] = {
{1004, "AAAA", 90},
{1002, "BBBB", 80},
{1005, "CCCC", 85},
{1007, "DDDD", 75},
{1001, "EEE", 65}
};
// 使用set
set<Student> mySet(stu, stu + 5);
for (const auto& s : mySet) {
cout << s.ID << " " << s.Name << " " << s.Score << endl;
}
// 使用multiset
multiset<Student> myMultiSet(stu, stu + 5);
for (const auto& s : myMultiSet) {
cout << s.ID << " " << s.Name << " " << s.Score << endl;
}
// 使用unordered_set
unordered_set<Student> myUnorderedSet(stu, stu + 5);
for (const auto& s : myUnorderedSet) {
cout << s.ID << " " << s.Name << " " << s.Score << endl;
}
return 0;
}
```
输出结果:
```
1001 EEE 65
1002 BBBB 80
1004 AAAA 90
1005 CCCC 85
1007 DDDD 75
1001 EEE 65
1002 BBBB 80
1004 AAAA 90
1005 CCCC 85
1007 DDDD 75
1004 AAAA 90
1001 EEE 65
1007 DDDD 75
1002 BBBB 80
1005 CCCC 85
```
可以看到,使用set、multiset和unordered_set都可以生成集合,并以学号ID为Key。其中,set和multiset会根据默认的比较函数operator<对元素进行排序,而unordered_set不会排序。
相关推荐
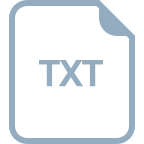
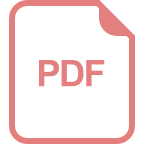














