c++ 正则表达式去除string中除字母之外的其他字符
时间: 2023-07-09 07:55:52 浏览: 93
您可以使用 C++11 引入的正则表达式库 `regex` 来完成该任务。下面是一个示例代码:
```c++
#include <iostream>
#include <string>
#include <regex>
int main() {
std::string str = "abc123def456";
std::regex reg("[^a-zA-Z]+");
str = std::regex_replace(str, reg, "");
std::cout << str << std::endl;
return 0;
}
```
在上面的代码中,首先定义了一个字符串 `str`,其中包含了字母和数字。然后使用正则表达式 `[^a-zA-Z]+` 匹配除字母之外的任何字符,其中 `^` 表示取反,`a-zA-Z` 表示匹配所有字母,`+` 表示匹配一个或多个字符。接着使用 `regex_replace` 函数将匹配到的字符替换为空字符串,最后输出结果。
输出结果为:
```
abcdef
```
可以看到,所有的数字都被成功地去除了,只剩下了字母。
相关问题
c++正则表达式
在 C++ 中,可以使用正则表达式库 `<regex>` 来实现正则表达式的功能。下面是一个简单的示例代码:
```cpp
#include <iostream>
#include <regex>
#include <string>
int main() {
std::string input_string = "Hello, world!";
std::regex pattern("world");
if (std::regex_search(input_string, pattern)) {
std::cout << "The pattern is found in the input string." << std::endl;
} else {
std::cout << "The pattern is not found in the input string." << std::endl;
}
return 0;
}
```
在上面的代码中,我们使用了 `<regex>` 库中的 `std::regex_search` 函数来搜索字符串中是否包含指定的模式。该函数的第一个参数是要搜索的字符串,第二个参数是正则表达式模式。如果找到了匹配的模式,则函数返回 true,否则返回 false。
除了 `std::regex_search` 函数之外,还有其他的正则表达式函数,比如 `std::regex_match`、`std::regex_replace` 等。详情可以参考 C++ 标准库的文档。
c++正则表达式字符串匹配
在 C++ 中,可以使用 <regex> 头文件中的正则表达式库来进行字符串匹配。具体步骤如下:
1. 定义正则表达式模式,使用 std::regex 类型表示。
2. 使用 std::regex_search() 或 std::regex_match() 函数进行匹配。
下面是一个简单的示例代码,演示如何使用正则表达式库进行字符串匹配:
```cpp
#include <iostream>
#include <regex>
int main() {
std::string str = "Hello, world!";
std::regex pattern("world");
if (std::regex_search(str, pattern)) {
std::cout << "Match found!" << std::endl;
} else {
std::cout << "Match not found." << std::endl;
}
return 0;
}
```
输出结果为:
```
Match found!
```
阅读全文
相关推荐
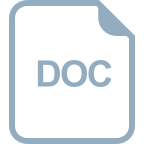
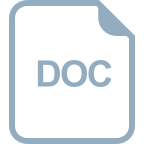
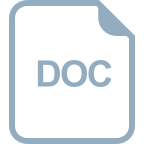
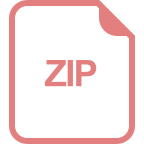


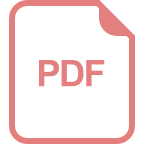









