优化一下代码package Y; import java.io.*; import java.net.*; import java.util.*; public class Shiyan5Client { public static void main(String[] args) { Scanner sc = new Scanner(System.in); Socket mysocket = null; DataInputStream in = null; DataOutputStream out = null; Thread timeData; TellTime time = null; try { mysocket = new Socket(); time = new TellTime(); timeData = new Thread(time); System.out.println("输入服务器IP:"); String IP = sc.nextLine(); System.out.println("输入端口号:"); int port = sc.nextInt(); if (mysocket.isConnected()) {} else { InetAddress address = InetAddress.getByName(IP); InetSocketAddress socketAddress = new InetSocketAddress(IP, port); mysocket.connect(socketAddress); in = new DataInputStream(mysocket.getInputStream()); out = new DataOutputStream(mysocket.getOutputStream()); time.setDataInputStream(in); timeData.start(); } } catch (Exception e) { System.out.println("服务器已断开:"+e); // TODO: handle exception } System.out.println("请输入服务:"); while (sc.hasNext()) { try { String service = sc.next(); out.writeUTF(service); } catch (Exception e) { // TODO: handle exception } } } }
时间: 2024-01-09 17:05:07 浏览: 81
可以尝试对代码进行以下优化:
1. 将输入输出流的初始化放在连接成功时进行,而非在Socket创建时进行,这样可以避免在连接失败时无法关闭输入输出流的情况。
2. 对于Thread的启动,建议使用ExecutorService来管理,这样可以更好地控制线程的启动和关闭。
3. 在异常处理中,可以添加一些具体的处理逻辑,比如关闭Socket等资源,避免资源泄露。
下面是优化后的代码:
```java
package Y;
import java.io.*;
import java.net.*;
import java.util.*;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class Shiyan5Client {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
Socket mysocket = null;
DataInputStream in = null;
DataOutputStream out = null;
ExecutorService executor = Executors.newSingleThreadExecutor();
TellTime time = null;
try {
System.out.println("输入服务器IP:");
String IP = sc.nextLine();
System.out.println("输入端口号:");
int port = sc.nextInt();
mysocket = new Socket();
InetAddress address = InetAddress.getByName(IP);
InetSocketAddress socketAddress = new InetSocketAddress(address, port);
mysocket.connect(socketAddress);
in = new DataInputStream(mysocket.getInputStream());
out = new DataOutputStream(mysocket.getOutputStream());
time = new TellTime();
time.setDataInputStream(in);
executor.execute(time);
} catch (Exception e) {
System.out.println("服务器已断开:" + e);
// TODO: handle exception
if (mysocket != null) {
try {
mysocket.close();
} catch (IOException ioException) {
ioException.printStackTrace();
}
}
if (in != null) {
try {
in.close();
} catch (IOException ioException) {
ioException.printStackTrace();
}
}
if (out != null) {
try {
out.close();
} catch (IOException ioException) {
ioException.printStackTrace();
}
}
executor.shutdown();
}
System.out.println("请输入服务:");
while (sc.hasNext()) {
try {
String service = sc.next();
out.writeUTF(service);
} catch (Exception e) {
// TODO: handle exception
if (mysocket != null) {
try {
mysocket.close();
} catch (IOException ioException) {
ioException.printStackTrace();
}
}
if (in != null) {
try {
in.close();
} catch (IOException ioException) {
ioException.printStackTrace();
}
}
if (out != null) {
try {
out.close();
} catch (IOException ioException) {
ioException.printStackTrace();
}
}
executor.shutdown();
}
}
}
}
```
阅读全文
相关推荐
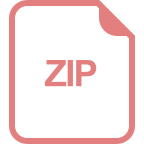
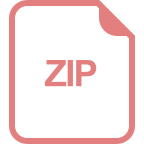
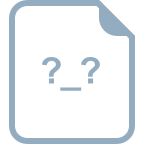
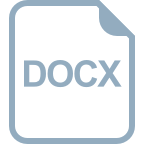
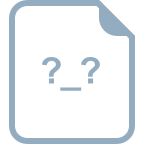
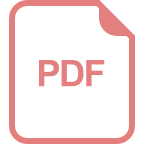
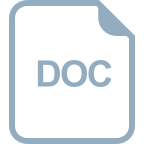
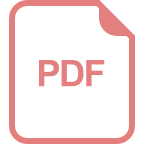
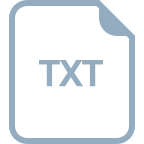
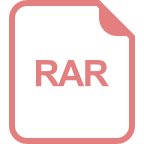
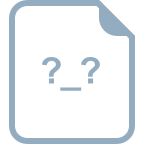
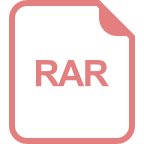
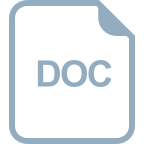
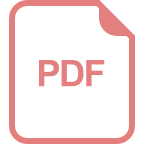
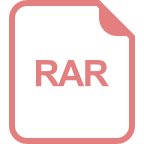
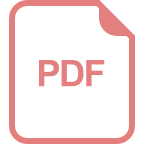
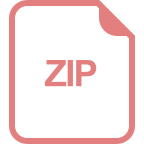