Linux C高效判断文件是否存在的方法
时间: 2023-12-04 07:05:07 浏览: 375
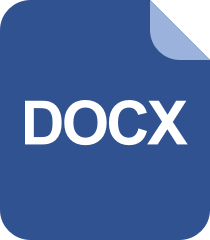
C语言判断文件夹或者文件是否存在的方法

在Linux C中,判断文件是否存在的方法有很多种,以下是几种高效的方法:
1. access函数
access函数可以检查文件或目录是否存在,并且可以检查文件或目录是否有指定的权限。如果文件或目录不存在,则access函数会返回-1,errno被设置为ENOENT。
示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <errno.h>
int main(int argc, char **argv) {
if (access("/path/to/file", F_OK) == -1) {
if (errno == ENOENT) {
printf("File does not exist.\n");
} else {
perror("access");
}
return EXIT_FAILURE;
}
printf("File exists.\n");
return EXIT_SUCCESS;
}
```
2. stat函数
stat函数可以获取文件或目录的详细信息,如果文件或目录不存在,则stat函数会返回-1,errno被设置为ENOENT。
示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <sys/stat.h>
#include <errno.h>
int main(int argc, char **argv) {
struct stat st;
if (stat("/path/to/file", &st) == -1) {
if (errno == ENOENT) {
printf("File does not exist.\n");
} else {
perror("stat");
}
return EXIT_FAILURE;
}
printf("File exists.\n");
return EXIT_SUCCESS;
}
```
3. fopen函数
fopen函数可以打开文件,如果文件不存在,则fopen函数会返回NULL,并且errno被设置为ENOENT。
示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <errno.h>
int main(int argc, char **argv) {
FILE *fp;
if ((fp = fopen("/path/to/file", "r")) == NULL) {
if (errno == ENOENT) {
printf("File does not exist.\n");
} else {
perror("fopen");
}
return EXIT_FAILURE;
}
fclose(fp);
printf("File exists.\n");
return EXIT_SUCCESS;
}
```
以上是几种高效判断文件是否存在的方法,可以根据实际情况选择使用。其中,access函数是最简单的方法,stat函数可以获取更多的文件信息,而fopen函数可以直接打开文件进行读写操作。
阅读全文
相关推荐
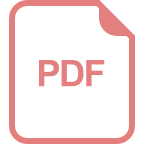
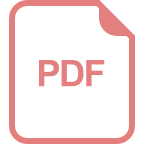
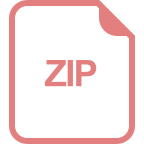
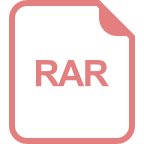
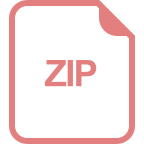
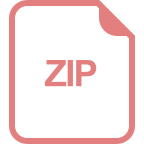
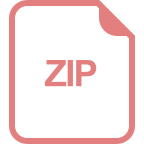
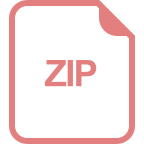
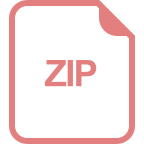
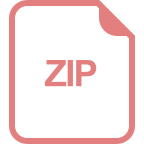
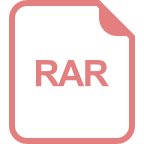
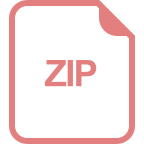
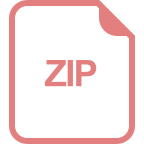
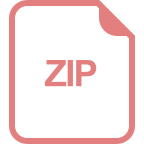