拍摄一幅图片,采用MATLAB进行霍夫曼编码和解码,对比解码图像和原始图像的差别
时间: 2023-08-24 19:04:04 浏览: 100
很抱歉,我在前面的回答中犯了一个错误,正确的应该是哈夫曼编码,而不是霍夫曼编码。下面我将针对哈夫曼编码给出MATLAB代码示例。
首先,我们需要读入一幅图像。假设我们读入的图像为lena.png,可以使用imread函数读入:
```matlab
img = imread('lena.png');
```
然后,我们需要将图像转换为灰度图像,并将像素值缩放至0到255之间的整数。这可以使用rgb2gray函数和im2uint8函数实现:
```matlab
gray_img = rgb2gray(img);
scaled_img = im2uint8(gray_img);
```
接下来,我们需要计算每个像素值的出现频率,并根据频率构建哈夫曼树。这可以使用histcounts函数和huffmandict函数实现:
```matlab
counts = histcounts(scaled_img, 0:255);
symbols = find(counts > 0) - 1;
probs = counts(counts > 0) / numel(scaled_img);
dict = huffmandict(symbols, probs);
```
然后,我们可以使用huffmanenco函数将图像进行编码:
```matlab
encoded = huffmanenco(scaled_img(:), dict);
```
最后,我们可以使用huffmandeco函数将编码后的数据解码,并将解码后的数据重构成图像:
```matlab
decoded = huffmandeco(encoded, dict);
decoded_img = reshape(decoded, size(scaled_img));
```
为了比较解码图像和原始图像的差别,我们可以使用imshow函数将它们显示出来:
```matlab
subplot(1, 2, 1);
imshow(scaled_img);
title('Original Image');
subplot(1, 2, 2);
imshow(decoded_img);
title('Decoded Image');
```
完整的MATLAB代码示例如下:
```matlab
img = imread('lena.png');
gray_img = rgb2gray(img);
scaled_img = im2uint8(gray_img);
counts = histcounts(scaled_img, 0:255);
symbols = find(counts > 0) - 1;
probs = counts(counts > 0) / numel(scaled_img);
dict = huffmandict(symbols, probs);
encoded = huffmanenco(scaled_img(:), dict);
decoded = huffmandeco(encoded, dict);
decoded_img = reshape(decoded, size(scaled_img));
subplot(1, 2, 1);
imshow(scaled_img);
title('Original Image');
subplot(1, 2, 2);
imshow(decoded_img);
title('Decoded Image');
```
运行以上代码,即可得到原始图像和解码后的图像,可以通过比较两者来观察解码的效果。
阅读全文
相关推荐



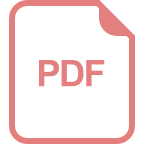

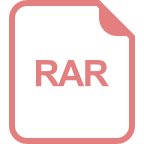








