编写一个coco数据集格式转voc数据集格式的python代码
时间: 2023-03-03 21:47:54 浏览: 155
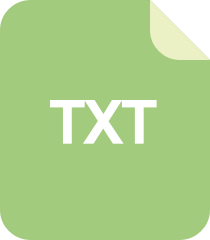
coco转为voc代码
以下是一个将COCO数据集格式转换为VOC数据集格式的Python代码示例:
```python
import os
import shutil
import json
import xml.etree.ElementTree as ET
from PIL import Image
# COCO数据集路径
coco_path = '/path/to/coco/dataset/'
# VOC数据集路径
voc_path = '/path/to/voc/dataset/'
# 创建VOC数据集所需的文件夹
if not os.path.exists(voc_path):
os.makedirs(voc_path)
if not os.path.exists(os.path.join(voc_path, 'Annotations')):
os.makedirs(os.path.join(voc_path, 'Annotations'))
if not os.path.exists(os.path.join(voc_path, 'JPEGImages')):
os.makedirs(os.path.join(voc_path, 'JPEGImages'))
if not os.path.exists(os.path.join(voc_path, 'ImageSets')):
os.makedirs(os.path.join(voc_path, 'ImageSets', 'Main'))
# 读取COCO数据集标注文件
with open(os.path.join(coco_path, 'annotations.json'), 'r') as f:
annotations = json.load(f)
# 处理每个图像
for image in annotations['images']:
# 读取图像
image_file = os.path.join(coco_path, 'images', image['file_name'])
im = Image.open(image_file)
# 保存图像
im.save(os.path.join(voc_path, 'JPEGImages', image['file_name']))
# 创建XML文件
xml_file = os.path.join(voc_path, 'Annotations', os.path.splitext(image['file_name'])[0] + '.xml')
root = ET.Element('annotation')
ET.SubElement(root, 'folder').text = 'VOC2012'
ET.SubElement(root, 'filename').text = image['file_name']
ET.SubElement(root, 'segmented').text = '0'
# 处理每个标注
for annotation in annotations['annotations']:
if annotation['image_id'] == image['id']:
# 添加对象信息
obj = ET.SubElement(root, 'object')
ET.SubElement(obj, 'name').text = annotation['category_id']
ET.SubElement(obj, 'pose').text = 'Unspecified'
ET.SubElement(obj, 'truncated').text = '0'
ET.SubElement(obj, 'difficult').text = '0'
bbox = annotation['bbox']
bndbox = ET.SubElement(obj, 'bndbox')
ET.SubElement(bndbox, 'xmin').text = str(bbox[0])
ET.SubElement(bndbox, 'ymin').text = str(bbox[1])
ET.SubElement(bndbox, 'xmax').text = str(bbox[0] + bbox[2])
ET.SubElement(bndbox, 'ymax').text = str(bbox[1] + bbox[3])
# 保存XML文件
tree = ET.ElementTree(root)
tree.write(xml_file)
# 创建ImageSets/Main/trainval.txt文件
with open(os.path.join(voc_path, 'ImageSets', 'Main', 'trainval.txt'), 'w') as f:
for image in annotations['images']:
f.write(os.path.splitext(image['file_name'])[0] + '\n')
# 将VOC数据集格式复制到COCO数据集所在目录
shutil.copytree(voc_path, os.path.join(coco_path, 'voc_dataset'))
```
该代码会读取COCO数据集的annotations.json文件,并将其中的每个图像转换为VOC数据
阅读全文
相关推荐
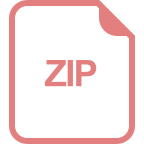
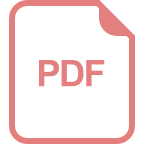
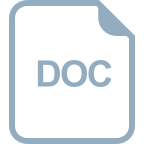
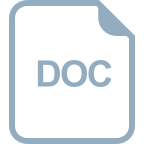

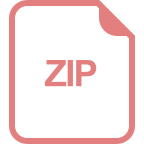
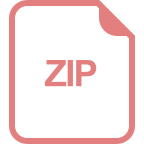
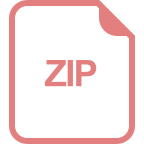
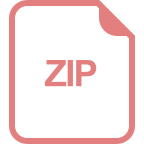
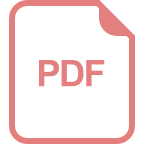
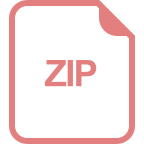
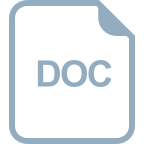
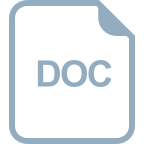
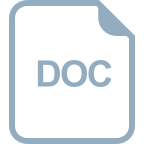
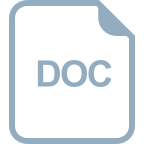
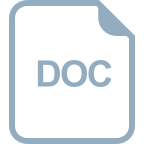
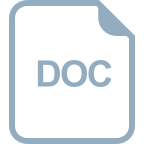
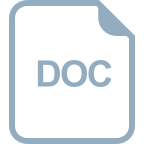