Map<String, List<StockTackingExportVO>> pnDetailMap = new HashMap<>(); for (StockTackingExportVO detail : pnDetail) { String pnCode = detail.getPnCode(); //在map中获取当前code,如果存在就是空数组, List<StockTackingExportVO> detailList = pnDetailMap.getOrDefault(pnCode, new ArrayList<>()); detailList.add(detail); pnDetailMap.put(pnCode, detailList); } 解释下代码的以上
时间: 2024-04-28 13:23:36 浏览: 171
这段代码的作用是将查询出来的 `pnDetail` 列表按照 PN 编码进行分组,每个 PN 编码对应一个 `StockTackingExportVO` 列表。
具体来说,代码的执行过程如下:
1. 创建一个空的 HashMap,用于保存 PN 编码和对应的 `StockTackingExportVO` 列表。
2. 遍历查询出来的 `pnDetail` 列表,对于每个 `StockTackingExportVO`,获取其 PN 编码。
3. 在 `pnDetailMap` 中获取当前 PN 编码对应的 `StockTackingExportVO` 列表,如果该列表不存在,则创建一个空的列表。
4. 将当前 `StockTackingExportVO` 添加到 PN 编码对应的列表中。
5. 将更新后的 PN 编码和对应的 `StockTackingExportVO` 列表保存到 `pnDetailMap` 中。
6. 遍历结束后,`pnDetailMap` 中保存了每个 PN 编码对应的 `StockTackingExportVO` 列表。
需要注意的是,这里使用了 `pnDetailMap.getOrDefault(pnCode, new ArrayList<>())` 方法来获取 PN 编码对应的列表,如果该列表不存在,则返回一个空的列表。这样可以避免空指针异常。
相关问题
优化这段代码:private List<DynamicSource> getDynamicSourceList(List<DasymesQltyMpCertfInfoItemMod> itemModList) { List<Map<String, String>> mapList = new ArrayList<>(); if (itemModList == null || itemModList.size() == 0) { Map<String, String> map = new HashMap<>(); map.put("no", null); map.put("name", null); map.put("portion", null); mapList.add(map); return DynamicSource.createList("detail", mapList); } for (int i = 1; i <= itemModList.size(); i++) { DasymesQltyMpCertfInfoItemMod item = itemModList.get(i - 1); Map<String, String> map = new HashMap<>(); map.put("no", i + ""); map.put("name", item.getItemName()); map.put("portion", item.getPortion()); mapList.add(map); } return DynamicSource.createList("detail", mapList); }
可以使用Java 8的Stream API来重写这段代码,代码如下:
```
private List<DynamicSource> getDynamicSourceList(List<DasymesQltyMpCertfInfoItemMod> itemModList) {
List<Map<String, String>> mapList = Optional.ofNullable(itemModList)
.orElseGet(Collections::emptyList)
.stream()
.map(item -> {
Map<String, String> map = new HashMap<>();
map.put("no", String.valueOf(itemModList.indexOf(item) + 1));
map.put("name", item.getItemName());
map.put("portion", item.getPortion());
return map;
})
.collect(Collectors.toList());
if (mapList.isEmpty()) {
Map<String, String> map = new HashMap<>();
map.put("no", null);
map.put("name", null);
map.put("portion", null);
mapList.add(map);
}
return DynamicSource.createList("detail", mapList);
}
```
这里使用了Optional来避免对空List进行null检查,使用Stream API来处理List数据并返回一个新的List。同时将代码中的循环和判断合并到了一起,使代码更加简洁易读。
优化这段代码: // 编辑动态数据 private List<DynamicSource> getDynamicSourceList(List<DasymesQltyMpCertfInfoItemMod> itemModList) { List<Map<String, String>> mapList = new ArrayList<>(); if (itemModList == null || itemModList.size() == 0) { Map<String, String> map = new HashMap<>(); map.put("no", null); map.put("name", null); map.put("portion", null); mapList.add(map); return DynamicSource.createList("detail", mapList); } for (int i = 1; i <= itemModList.size(); i++) { DasymesQltyMpCertfInfoItemMod item = itemModList.get(i - 1); Map<String, String> map = new HashMap<>(); map.put("no", i + ""); map.put("name", item.getItemName()); map.put("portion", item.getPortion()); mapList.add(map); } return DynamicSource.createList("detail", mapList); }
这段代码的主要作用是将 `itemModList` 转换为 `DynamicSource` 类型的数据。优化的点主要集中在两个方面:一是代码的可读性,二是代码的性能。
以下是优化后的代码:
```
private List<DynamicSource> getDynamicSourceList(List<DasymesQltyMpCertfInfoItemMod> itemModList) {
List<Map<String, String>> mapList = new ArrayList<>();
if (itemModList == null || itemModList.isEmpty()) {
mapList.add(Collections.emptyMap());
} else {
for (int i = 0; i < itemModList.size(); i++) {
DasymesQltyMpCertfInfoItemMod item = itemModList.get(i);
Map<String, String> map = new HashMap<>();
map.put("no", String.valueOf(i + 1));
map.put("name", item.getItemName());
map.put("portion", item.getPortion());
mapList.add(map);
}
}
return DynamicSource.createList("detail", mapList);
}
```
针对可读性方面的优化:
1. 将判断空列表的代码从 `if (itemModList == null || itemModList.size() == 0)` 修改为 `if (itemModList == null || itemModList.isEmpty())`,更加简洁易懂。
2. 在空列表的情况下,使用 `Collections.emptyMap()` 创建一个空的 Map 对象,代替手动创建一个 Map 对象,并将其中的属性设为 null。
3. 将 `for` 循环的起始索引从 1 修改为 0,更符合 Java 语言的习惯。
4. 将 `i + ""` 修改为 `String.valueOf(i + 1)`,更加规范。
针对性能方面的优化:
1. 使用 `isEmpty()` 方法判断列表是否为空,避免调用 `size()` 方法造成不必要的性能开销。
2. 使用增强型的 `for` 循环代替传统的 `for` 循环,代码简洁易懂,性能也更好。
3. 在空列表的情况下,仅创建一个空的 Map 对象,避免创建不必要的对象。
综上所述,通过上述优化,代码变得更加简洁易懂,同时也提高了代码的性能。
阅读全文
相关推荐
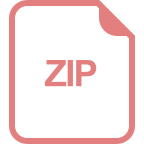
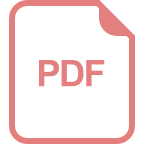
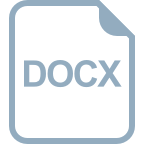

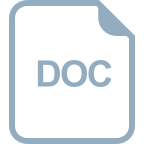
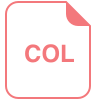
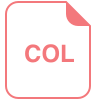
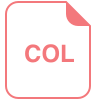
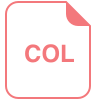
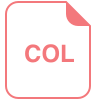
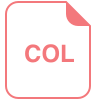



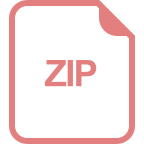