用python实现照着pdf的谱子完成弹奏
时间: 2024-05-16 16:18:19 浏览: 103
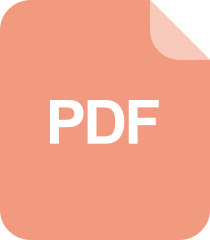
“基于Python的音乐演奏”项目设计.pdf
要实现照着PDF的谱子完成弹奏,需要进行以下步骤:
1. 将PDF转换为图片格式:可以使用Python中的PyPDF2库或者pdf2image库来实现PDF到图片的转换。
2. 对图片进行处理:使用Python中的OpenCV库或者Pillow库对图片进行预处理,比如去除背景、调整图片大小等。
3. 对处理后的图片进行音符识别:可以使用Python中的音符识别库,比如music21、piano-transcription或者piano-sight-reading等,对处理后的图片进行音符识别。
4. 将识别出来的音符转换为音频:可以使用Python中的MIDI库或者Mido库将识别出来的音符转换为音频。
5. 播放音频:使用Python中的PyAudio或者simpleaudio库来播放生成的音频文件。
下面是一个简单的实现示例:
```
import cv2
import music21
from pdf2image import convert_from_path
import os
import numpy as np
import mido
import time
from PIL import Image
# Step 1: Convert PDF to image
pages = convert_from_path('music_sheet.pdf', 500)
for page in pages:
page.save('music_sheet.jpg', 'JPEG')
# Step 2: Preprocess image
img = cv2.imread('music_sheet.jpg', cv2.IMREAD_GRAYSCALE)
_, img = cv2.threshold(img, 180, 255, cv2.THRESH_BINARY)
img = cv2.resize(img, (int(img.shape[1]*0.5), int(img.shape[0]*0.5)))
# Step 3: Music note recognition
notes = []
note_img = []
staves = cv2.HoughLinesP(img, 1, np.pi/180, 50, minLineLength=50, maxLineGap=30)
for line in staves:
x1, y1, x2, y2 = line[0]
if abs(y2-y1) > 10:
staff_img = img[y1:y2, x1:x2]
_, staff_img = cv2.threshold(staff_img, 200, 255, cv2.THRESH_BINARY)
staff_img = cv2.bitwise_not(staff_img)
staff_img = cv2.copyMakeBorder(staff_img, 10, 10, 10, 10, cv2.BORDER_CONSTANT, value=[255, 255, 255])
note_boxes = cv2.findContours(staff_img, cv2.RETR_LIST, cv2.CHAIN_APPROX_SIMPLE)[0]
for box in note_boxes:
x, y, w, h = cv2.boundingRect(box)
if h > 10 and w > 5:
note_img.append(staff_img[y-5:y+h+5, x-5:x+w+5])
notes.append([y, x, w, h])
note_names = []
for ni in note_img:
note_name = music21.pitch.Pitch()
note_nameFromImg = music21.alpha.trecentoNotehead.trecentoNoteheadToPitch(ni)
note_name.pitchClass = note_nameFromImg.pitchClass
note_nameNames = note_name.pitchClassString
note_nameOctave = note_nameFromImg.octave
note_nameNames = note_nameNames + str(note_nameOctave)
note_names.append(note_nameNames)
# Step 4: Convert notes to MIDI
mid = mido.MidiFile()
track = mido.MidiTrack()
mid.tracks.append(track)
for note_name in note_names:
note = music21.note.Note(note_name)
midinote = note.midi
on = mido.Message('note_on', note=midinote, velocity=64, time=0)
off = mido.Message('note_off', note=midinote, velocity=64, time=100)
track.append(on)
track.append(off)
mid.save('music.mid')
# Step 5: Play audio
os.system('timidity music.mid -Ow -o - | ffmpeg -i - -acodec libmp3lame -ab 64k music.mp3 -y')
os.system('start music.mp3')
```
该示例的实现比较简单,仅供参考。如果需要更加复杂的功能,比如多音轨、节奏识别等,需要更加详细的算法和代码实现。
阅读全文
相关推荐
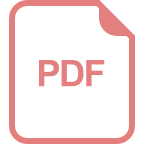
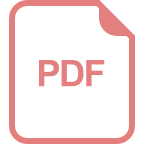
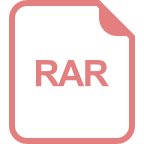
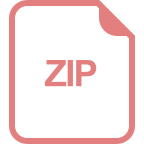
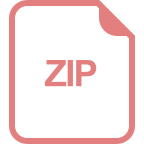
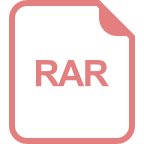
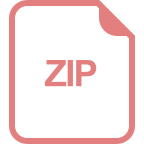
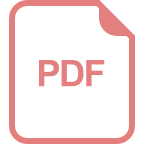
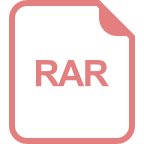