使用C语言去掉字符串中的换行符
时间: 2023-12-03 08:43:22 浏览: 517
可以使用C语言中的字符串处理函数 `strchr()` 和 `strcpy()` 去掉字符串中的换行符,具体实现如下:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[100];
printf("输入包含换行符的字符串:\n");
fgets(str, sizeof(str), stdin);
char *pos = strchr(str, '\n'); // 查找换行符的位置
if (pos != NULL) {
*pos = '\0'; // 将换行符替换成字符串结束符
}
printf("去掉换行符后的字符串为:%s\n", str);
return 0;
}
```
在上述代码中,`fgets()` 函数用于从标准输入中读取字符串,包含换行符。然后使用 `strchr()` 函数查找字符串中的换行符,并将其替换成字符串结束符 `\0`。最后输出去掉换行符的字符串。
相关问题
C语言如何去掉字符串中的换行符和回车符
在 C 语言中,可以使用字符串处理函数 `strcspn`、`strchr` 和 `strcpy` 来去掉字符串中的换行符和回车符。
具体步骤如下:
1. 使用 `strcspn` 函数找到字符串中第一个换行符或回车符的位置,它返回的是第一个匹配字符的位置。
2. 如果找到了换行符或回车符,则使用 `strcpy` 函数将该位置之后的字符全部复制到该位置,覆盖换行符或回车符。
3. 继续使用 `strcspn` 函数寻找下一个换行符或回车符的位置,直到字符串结束。
示例代码如下:
```c
#include <stdio.h>
#include <string.h>
void remove_newline(char str[]) {
char *pos;
while ((pos = strchr(str, '\n')) != NULL || (pos = strchr(str, '\r')) != NULL) {
strcpy(pos, pos+1);
}
}
int main() {
char str[100] = "Hello, world!\nThis is a test.\r\n";
printf("Before: %s", str);
remove_newline(str);
printf("After: %s", str);
return 0;
}
```
输出结果为:
```
Before: Hello, world!
This is a test.
After: Hello, world!This is a test.
```
c++如和去掉字符串中的换行符
### 回答1:
C 语言中,通常使用字符串函数 `strtok` 来删除字符串中的换行符。下面是一个例子:
```
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello,\nworld!\n";
char *p;
p = strtok(str, "\n");
while (p != NULL) {
printf("%s ", p);
p = strtok(NULL, "\n");
}
return 0;
}
```
运行该程序,输出结果为:
```
Hello, world!
```
可以看到,换行符已被删除。
### 回答2:
在C语言中,可以通过遍历字符串的每一个字符,判断是否为换行符'\n',如果是的话将其去掉。具体的实现可以通过如下代码来实现:
```c
#include <stdio.h>
#include <string.h>
void removeNewLine(char* str) {
int len = strlen(str); // 获取字符串的长度
for(int i = 0; i < len; i++) {
if(str[i] == '\n') { // 判断当前字符是否为换行符
for(int j = i; j < len; j++) {
// 将后面的字符向前移动一位,覆盖掉换行符
str[j] = str[j+1];
}
len--; // 字符串长度减1
i--; // 由于字符向前移动了一位,需要将i的值减1保持在原来的位置继续判断
}
}
}
int main() {
char str[] = "Hello\nWorld!\n"; // 待处理的字符串
printf("原始字符串:\n%s\n", str);
removeNewLine(str); // 去掉换行符
printf("去掉换行符后的字符串:\n%s\n", str);
return 0;
}
```
在上述代码中,我们定义了一个函数`removeNewLine`,通过遍历字符串并判断是否为换行符,如果是的话通过将后面的字符向前移动一位来去除换行符。最后在`main`函数中,我们定义一个待处理的字符串,然后调用`removeNewLine`函数来去掉换行符并打印出结果。
### 回答3:
在Python中,可以使用字符串的`replace()`方法去掉字符串中的换行符。该方法接受两个参数,第一个参数是要搜索的子字符串,第二个参数是要替换成的新字符串。我们可以将换行符"\n"作为第一个参数传入,并将空字符串""作为第二个参数传入。以下是一个示例代码:
```python
def remove_newlines(text):
new_text = text.replace("\n", "")
return new_text
text = "这是一个有\n换行符的\n字符串。"
result = remove_newlines(text)
print(result)
```
在上面的代码中,我们先定义了一个`remove_newlines`函数,接受一个参数`text`,表示要去掉换行符的字符串。函数内部使用`replace()`方法将换行符"\n"替换为空字符串"",并将结果保存在`new_text`变量中。最后,我们打印出`new_text`来查看去掉换行符后的字符串。
输出结果为:"这是一个有换行符的字符串。",可以看到字符串中的换行符已经被去掉了。
相关推荐
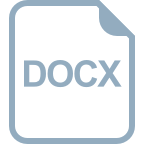
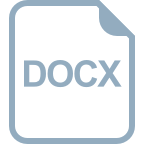
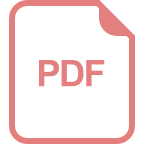












