Write a C++ program that implements flipping the contents of each line of a string in a text file and outputs the flipped result to another text file. Both the input file name and output file name are specified by the user. Example: Suppose the input file input.txt has the following contents: Hello, world! Welcome to C++! After executing the program, the output file output.txt should contain the following contents: !dlrow ,olleH !++C ot emocleW Tip: You can use the getline() function to read the string from the input file line by line, then use the reverse() function to flip the string, and finally write the flipped string to the output file.
时间: 2024-03-01 21:53:29 浏览: 24
Sure, here's a C++ program that implements flipping the contents of each line of a string in a text file and outputs the flipped result to another text file:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <algorithm>
using namespace std;
int main() {
string inputFileName, outputFileName;
cout << "Enter input file name: ";
cin >> inputFileName;
cout << "Enter output file name: ";
cin >> outputFileName;
ifstream inputFile(inputFileName);
if (!inputFile.is_open()) {
cout << "Error opening input file." << endl;
return 1;
}
ofstream outputFile(outputFileName);
if (!outputFile.is_open()) {
cout << "Error opening output file." << endl;
return 1;
}
string line;
while (getline(inputFile, line)) {
reverse(line.begin(), line.end());
outputFile << line << endl;
}
inputFile.close();
outputFile.close();
cout << "File contents flipped successfully." << endl;
return 0;
}
```
In this program, we first ask the user to enter the input file name and output file name. We then open the input file using ifstream and check if the file was opened successfully. Similarly, we open the output file using ofstream and check if the file was opened successfully.
We then read the input file line by line using getline(), and flip each line using the reverse() function from the algorithm library. Finally, we write the flipped line to the output file using ofstream.
After all the lines have been flipped and written to the output file, we close both the input and output files and display a success message.
Note that this program assumes that the input file contains valid text data and that the output file can be created or overwritten without any issues.
相关推荐
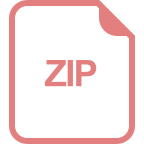















