遗传算法求解多元函数最小值的代码是
时间: 2023-11-19 10:52:52 浏览: 25
以下是一个简单的遗传算法求解多元函数最小值的 Python 代码示例:
```python
import numpy as np
# 定义目标函数,这里以二元函数 f(x,y) = x^2 + y^2 为例
def objective_function(x):
return x[0]**2 + x[1]**2
# 定义遗传算法函数
def genetic_algorithm(objective_function, bounds, population_size=100, max_generations=100, mutation_probability=0.1):
# 初始化种群
population = np.random.rand(population_size, len(bounds)) * (bounds[:, 1] - bounds[:, 0]) + bounds[:, 0]
# 迭代
for i in range(max_generations):
# 计算适应度
fitness = np.array([objective_function(ind) for ind in population])
# 找到最佳个体
best_individual = population[np.argmin(fitness)]
# 输出当前迭代次数和最佳个体的适应度
print("Generation {}: Best fitness = {:.4f}".format(i+1, objective_function(best_individual)))
# 选择
parents = np.empty((population_size, len(bounds)))
for j in range(population_size):
# 轮盘赌选择
idx = np.random.choice(population_size, size=2, replace=False, p=fitness/np.sum(fitness))
parents[j] = population[idx[0]]
# 交叉
children = np.empty_like(parents)
for j in range(0, population_size, 2):
# 单点交叉
point = np.random.randint(1, len(bounds))
children[j] = np.concatenate((parents[j, :point], parents[j+1, point:]))
children[j+1] = np.concatenate((parents[j+1, :point], parents[j, point:]))
# 变异
for j in range(population_size):
# 每个维度上都有 mutation_probability 的概率进行变异
if np.random.rand() < mutation_probability:
point = np.random.randint(0, len(bounds))
children[j, point] = np.random.uniform(bounds[point, 0], bounds[point, 1])
# 更新种群
population = children
# 返回最佳个体
return best_individual
# 测试
bounds = np.array([[-5.0, 5.0], [-5.0, 5.0]]) # 变量的范围
best_individual = genetic_algorithm(objective_function, bounds)
print("Best individual: ", best_individual)
print("Best fitness: ", objective_function(best_individual))
```
该代码使用轮盘赌选择、单点交叉和每个维度上都有一定概率进行变异的方式进行遗传算法优化,可以根据需要进行修改。
阅读全文
相关推荐
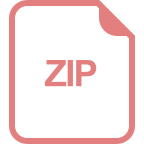
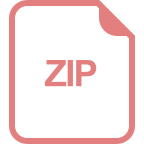

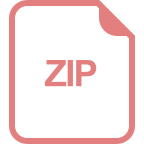
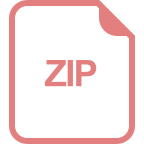
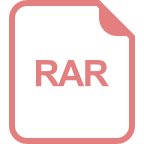
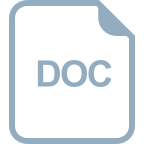
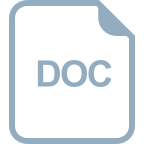
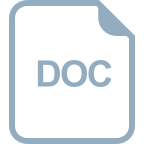
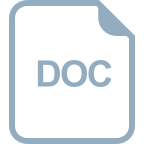

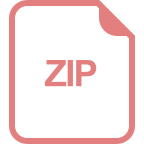
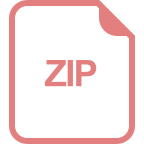
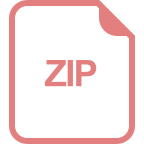
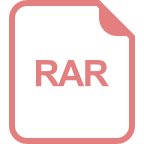