Android系统实现记录用户的开关机数据,获取某段时间内的用户开机率,并且将开关机数据以及用户开机率保存到日志文件中
时间: 2024-01-21 11:16:11 浏览: 148
Android系统提供了相应的API来获取设备的开关机数据,可以通过BroadcastReceiver接收设备开关机的广播,并在接收到广播时记录相应的数据。以下是一个示例代码:
```java
public class BootReceiver extends BroadcastReceiver {
private static final String TAG = "BootReceiver";
@Override
public void onReceive(Context context, Intent intent) {
if (intent == null) {
return;
}
String action = intent.getAction();
if (action == null) {
return;
}
switch (action) {
case Intent.ACTION_BOOT_COMPLETED:
// 设备开机
Log.i(TAG, "Boot completed");
saveBootData(context, true);
break;
case Intent.ACTION_SHUTDOWN:
// 设备关机
Log.i(TAG, "Shutdown");
saveBootData(context, false);
break;
}
}
private void saveBootData(Context context, boolean isBoot) {
SharedPreferences sp = context.getSharedPreferences("boot_data", Context.MODE_PRIVATE);
SharedPreferences.Editor editor = sp.edit();
String key = new SimpleDateFormat("yyyy-MM-dd", Locale.getDefault()).format(new Date());
int bootCount = sp.getInt(key + "_boot_count", 0);
int shutdownCount = sp.getInt(key + "_shutdown_count", 0);
if (isBoot) {
bootCount++;
} else {
shutdownCount++;
}
editor.putInt(key + "_boot_count", bootCount);
editor.putInt(key + "_shutdown_count", shutdownCount);
editor.apply();
}
}
```
可以在AndroidManifest.xml中注册该BroadcastReceiver:
```xml
<receiver android:name=".BootReceiver">
<intent-filter>
<action android:name="android.intent.action.BOOT_COMPLETED" />
<action android:name="android.intent.action.SHUTDOWN" />
</intent-filter>
</receiver>
```
可以使用JobScheduler或AlarmManager定时获取某段时间内的用户开机率,以下是一个示例代码:
```java
public class BootJobService extends JobService {
private static final String TAG = "BootJobService";
private static final int JOB_ID = 1001;
private static final long INTERVAL = 24 * 60 * 60 * 1000; // 一天
@Override
public boolean onStartJob(JobParameters params) {
Log.i(TAG, "onStartJob");
getBootRate(getApplicationContext());
scheduleNextJob();
return false;
}
@Override
public boolean onStopJob(JobParameters params) {
Log.i(TAG, "onStopJob");
return false;
}
private void scheduleNextJob() {
JobScheduler jobScheduler = (JobScheduler) getSystemService(Context.JOB_SCHEDULER_SERVICE);
if (jobScheduler == null) {
return;
}
JobInfo jobInfo = new JobInfo.Builder(JOB_ID, new ComponentName(this, BootJobService.class))
.setMinimumLatency(INTERVAL)
.setOverrideDeadline(INTERVAL + 60 * 1000) // 最多延迟一分钟
.setRequiresCharging(false)
.setPersisted(true)
.build();
jobScheduler.schedule(jobInfo);
}
private void getBootRate(Context context) {
SharedPreferences sp = context.getSharedPreferences("boot_data", Context.MODE_PRIVATE);
String key = new SimpleDateFormat("yyyy-MM-dd", Locale.getDefault()).format(new Date());
int bootCount = sp.getInt(key + "_boot_count", 0);
int shutdownCount = sp.getInt(key + "_shutdown_count", 0);
double rate = bootCount * 1.0 / (bootCount + shutdownCount);
Log.i(TAG, "Boot rate: " + rate);
// TODO: 将数据保存到日志文件中
}
}
```
可以在应用启动时启动定时任务:
```java
public class MainActivity extends AppCompatActivity {
private static final String TAG = "MainActivity";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
scheduleJob();
}
private void scheduleJob() {
JobScheduler jobScheduler = (JobScheduler) getSystemService(Context.JOB_SCHEDULER_SERVICE);
if (jobScheduler == null) {
return;
}
JobInfo jobInfo = new JobInfo.Builder(BootJobService.JOB_ID, new ComponentName(this, BootJobService.class))
.setMinimumLatency(BootJobService.INTERVAL)
.setOverrideDeadline(BootJobService.INTERVAL + 60 * 1000) // 最多延迟一分钟
.setRequiresCharging(false)
.setPersisted(true)
.build();
jobScheduler.schedule(jobInfo);
Log.i(TAG, "Job scheduled");
}
}
```
最后,可以使用File类将数据保存到日志文件中。
阅读全文
相关推荐
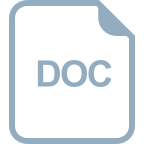
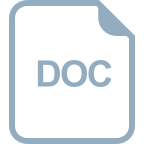
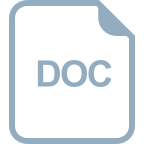
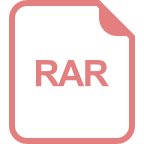
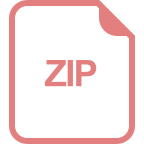
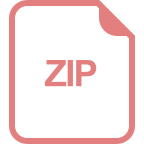
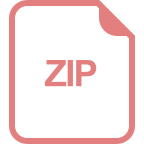
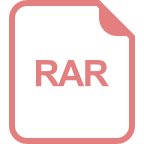
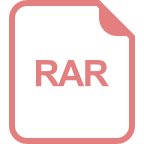
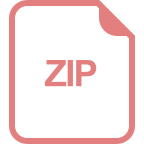
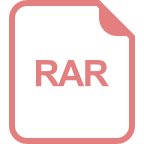
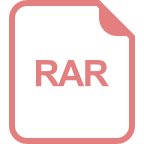
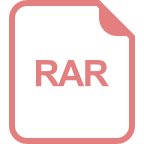
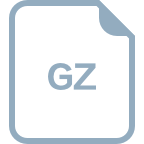
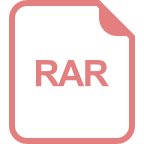
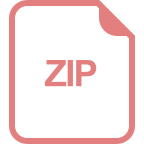
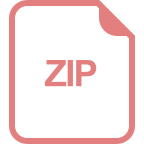
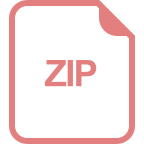