stm32串口1串口2同时收发数据
时间: 2023-09-12 11:03:31 浏览: 121
在 STM32 中,串口可以通过中断方式接收和发送数据,因此你可以同时使用串口1和串口2接收和发送数据。
下面是一个简单的示例代码,演示如何使用 STM32 的串口1和串口2 同时接收和发送数据。
```c
#include "stm32f10x.h"
#include <stdio.h>
#define UART1_BUFFER_SIZE 256
#define UART2_BUFFER_SIZE 256
volatile uint8_t uart1_rx_buffer[UART1_BUFFER_SIZE];
volatile uint8_t uart2_rx_buffer[UART2_BUFFER_SIZE];
volatile uint8_t uart1_rx_head = 0;
volatile uint8_t uart1_rx_tail = 0;
volatile uint8_t uart2_rx_head = 0;
volatile uint8_t uart2_rx_tail = 0;
void USART1_IRQHandler(void)
{
// check if the USART1 receive interrupt flag was set
if(USART_GetITStatus(USART1, USART_IT_RXNE) != RESET)
{
// read the received data
uint8_t rx_data = (uint8_t)(USART_ReceiveData(USART1) & 0xFF);
// store the received data in the buffer
uint8_t next_head = (uart1_rx_head + 1) % UART1_BUFFER_SIZE;
if(next_head != uart1_rx_tail)
{
uart1_rx_buffer[uart1_rx_head] = rx_data;
uart1_rx_head = next_head;
}
}
}
void USART2_IRQHandler(void)
{
// check if the USART2 receive interrupt flag was set
if(USART_GetITStatus(USART2, USART_IT_RXNE) != RESET)
{
// read the received data
uint8_t rx_data = (uint8_t)(USART_ReceiveData(USART2) & 0xFF);
// store the received data in the buffer
uint8_t next_head = (uart2_rx_head + 1) % UART2_BUFFER_SIZE;
if(next_head != uart2_rx_tail)
{
uart2_rx_buffer[uart2_rx_head] = rx_data;
uart2_rx_head = next_head;
}
}
}
void uart1_send_byte(uint8_t tx_data)
{
// wait for the transmit buffer to be empty
while(USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
// send the data
USART_SendData(USART1, tx_data);
}
void uart2_send_byte(uint8_t tx_data)
{
// wait for the transmit buffer to be empty
while(USART_GetFlagStatus(USART2, USART_FLAG_TXE) == RESET);
// send the data
USART_SendData(USART2, tx_data);
}
int main(void)
{
// configure GPIO pins for USART1 and USART2
// ...
// configure USART1 for 115200 baud, 8-bit data, no parity, 1 stop bit
USART_InitTypeDef usart1_config;
usart1_config.USART_BaudRate = 115200;
usart1_config.USART_WordLength = USART_WordLength_8b;
usart1_config.USART_StopBits = USART_StopBits_1;
usart1_config.USART_Parity = USART_Parity_No;
usart1_config.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
usart1_config.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &usart1_config);
// configure USART2 for 115200 baud, 8-bit data, no parity, 1 stop bit
USART_InitTypeDef usart2_config;
usart2_config.USART_BaudRate = 115200;
usart2_config.USART_WordLength = USART_WordLength_8b;
usart2_config.USART_StopBits = USART_StopBits_1;
usart2_config.USART_Parity = USART_Parity_No;
usart2_config.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
usart2_config.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART2, &usart2_config);
// enable USART1 and USART2 interrupts
USART_ITConfig(USART1, USART_IT_RXNE, ENABLE);
USART_ITConfig(USART2, USART_IT_RXNE, ENABLE);
NVIC_EnableIRQ(USART1_IRQn);
NVIC_EnableIRQ(USART2_IRQn);
// enable USART1 and USART2
USART_Cmd(USART1, ENABLE);
USART_Cmd(USART2, ENABLE);
while(1)
{
// check if there is any data in the USART1 receive buffer
while(uart1_rx_head != uart1_rx_tail)
{
// read the received data from the buffer
uint8_t rx_data = uart1_rx_buffer[uart1_rx_tail];
// process the received data
// ...
// move the tail pointer to the next position in the buffer
uart1_rx_tail = (uart1_rx_tail + 1) % UART1_BUFFER_SIZE;
}
// check if there is any data in the USART2 receive buffer
while(uart2_rx_head != uart2_rx_tail)
{
// read the received data from the buffer
uint8_t rx_data = uart2_rx_buffer[uart2_rx_tail];
// process the received data
// ...
// move the tail pointer to the next position in the buffer
uart2_rx_tail = (uart2_rx_tail + 1) % UART2_BUFFER_SIZE;
}
// send some data over USART1 and USART2
uart1_send_byte('h');
uart2_send_byte('i');
// wait for some time
// ...
}
}
```
在这个示例代码中,我们使用了两个缓冲区来存储串口1和串口2接收到的数据。当有数据到达时,中断会触发,将接收到的数据存储在相应的缓冲区中。在主循环中,我们不断检查缓冲区中是否有待处理的数据,并在需要时发送一些数据。请注意,我们在发送数据之前等待 USART 的发送缓冲区变为空闲状态。
阅读全文
相关推荐
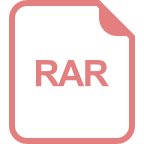
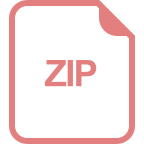
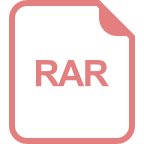
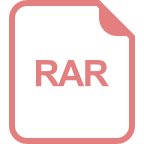
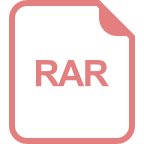
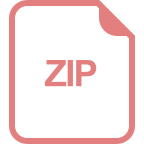
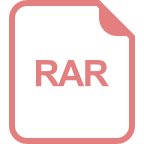
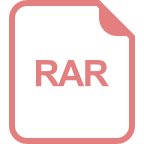
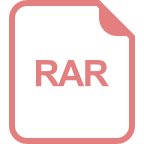
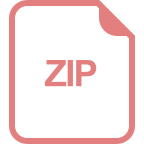
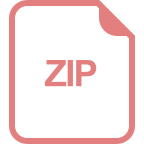
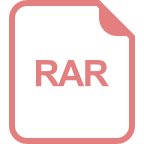
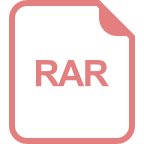
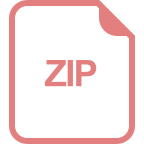