用c++定义一个能够交换两个字符串的函数
时间: 2023-12-16 18:06:27 浏览: 167
可以使用C++的string类来实现字符串交换,具体的代码如下:
```c++
#include <iostream>
#include <string>
using namespace std;
void swapString(string& str1, string& str2) {
string temp = str1;
str1 = str2;
str2 = temp;
}
int main() {
string str1 = "Hello";
string str2 = "World";
cout << "Before swap: str1 = " << str1 << ", str2 = " << str2 << endl;
swapString(str1, str2);
cout << "After swap: str1 = " << str1 << ", str2 = " << str2 << endl;
return 0;
}
```
在上述代码中,我们定义了一个名为`swapString`的函数,参数为两个字符串的引用。函数内部使用一个中间变量`temp`来存储`str1`的值,然后将`str1`赋值为`str2`,最后将`str2`赋值为`temp`,完成两个字符串的交换。在`main`函数中,我们调用了`swapString`函数来交换两个字符串,并输出交换后的结果。
相关问题
请定义一个能够交换两个字符串的函数(C++ string方法)。
好的,下面是一个能够交换两个字符串的函数的定义:
```c++
#include <string>
void swapStrings(std::string& str1, std::string& str2) {
std::string temp = str1;
str1 = str2;
str2 = temp;
}
```
使用方法如下:
```c++
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello";
std::string str2 = "World";
std::cout << "Before swapping: " << str1 << " " << str2 << std::endl;
swapStrings(str1, str2);
std::cout << "After swapping: " << str1 << " " << str2 << std::endl;
return 0;
}
```
输出结果为:
```
Before swapping: Hello World
After swapping: World Hello
```
希望这个函数能够满足你的需求!
、引用编程训练:请定义一个能够交换两个字符串的函数(C++ string方法)。
好的,下面是一个能够交换两个字符串的函数的定义:
```c++
#include <string>
void swapStrings(std::string& str1, std::string& str2) {
std::string temp = str1;
str1 = str2;
str2 = temp;
}
```
使用方法如下:
```c++
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello";
std::string str2 = "World";
std::cout << "Before swapping: " << str1 << " " << str2 << std::endl;
swapStrings(str1, str2);
std::cout << "After swapping: " << str1 << " " << str2 << std::endl;
return 0;
}
```
输出结果为:
```
Before swapping: Hello World
After swapping: World Hello
```
希望这个函数能够满足你的需求!
阅读全文
相关推荐
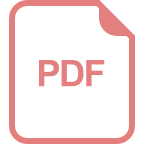

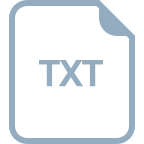
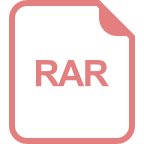
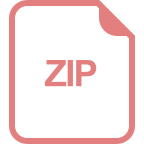
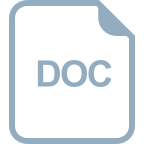
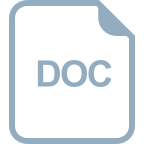
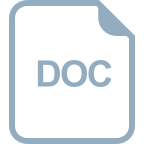






