首先,提示用户输入一个月份,将用户输入的值存放到变量 month 中; 然后,使用 switch 语句判断这个月份的天数(不考虑闰年); 最后,输出结果(例如:用户输入12,则系统输出:12月有31天)。
时间: 2023-05-30 10:06:34 浏览: 59
代码如下:
```python
month = int(input("请输入一个月份:"))
# 判断月份并输出天数
if month in [1, 3, 5, 7, 8, 10, 12]:
print(month, "月有31天")
elif month in [4, 6, 9, 11]:
print(month, "月有30天")
elif month == 2:
print(month, "月有28天")
else:
print("输入的月份不正确!")
```
使用 switch 语句实现:
```python
month = int(input("请输入一个月份:"))
# 使用 switch 语句判断月份并输出天数
switcher = {
1: "31天",
2: "28天",
3: "31天",
4: "30天",
5: "31天",
6: "30天",
7: "31天",
8: "31天",
9: "30天",
10: "31天",
11: "30天",
12: "31天"
}
days = switcher.get(month, "输入的月份不正确!")
print(month, "月有", days)
```
以上两种方法都可以实现获取输入月份的天数,第二种方法使用了字典和 switch 语句,代码更简洁,但需要先定义字典并预先处理好所有的月份,适用于月份较少的情况。
相关问题
首先,提示用户输入一个月份,将用户输入的值存放到变量 month 中; 然后,使用 switch 语句判断这个月份的天数(不考虑闰年); 最后,输出结果(例如:用户输入12,则系统输出:12月有31天)。
以下是Python实现:
month = int(input("请输入一个月份:"))
if month < 1 or month > 12:
print("输入的月份不合法")
else:
days = 0
if month == 2:
days = 28
elif month == 4 or month == 6 or month == 9 or month == 11:
days = 30
else:
days = 31
print("{}月有{}天".format(month, days))
以下是Java实现:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入一个月份:");
int month = scanner.nextInt();
scanner.close();
int days = 0;
switch (month) {
case 2:
days = 28;
break;
case 4:
case 6:
case 9:
case 11:
days = 30;
break;
default:
days = 31;
break;
}
System.out.printf("%d月有%d天", month, days);
}
}
编写程序,实现以下功能: 首先,提示用户输入一个月份,将用户输入的值存放到变量 month 中; 然后,使用 switch 语句判断这个月份的天数(不考虑闰年); 最后,输出结果(例如:用户输入12,则系统输出:12月有31天)。
```python
month = int(input("请输入一个月份:"))
days = 0
if month in [1, 3, 5, 7, 8, 10, 12]:
days = 31
elif month in [4, 6, 9, 11]:
days = 30
elif month == 2:
days = 28
print(f"{month}月有{days}天")
```
注:以上代码使用了 f-string,需要 Python3.6 及以上版本支持。如果使用的是 Python3.5 及以下版本,请使用以下代码:
```python
month = int(input("请输入一个月份:"))
days = 0
if month in [1, 3, 5, 7, 8, 10, 12]:
days = 31
elif month in [4, 6, 9, 11]:
days = 30
elif month == 2:
days = 28
print("{}月有{}天".format(month, days))
```
相关推荐
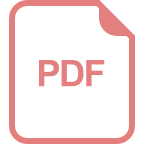
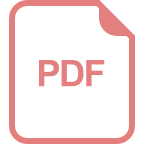
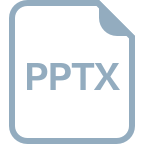












