C# 获取本地文件路径
时间: 2023-08-16 17:03:42 浏览: 58
在 C# 中,可以使用 OpenFileDialog 对话框来获取本地文件路径。示例代码如下:
```C#
// 创建 OpenFileDialog 对象
OpenFileDialog openFileDialog = new OpenFileDialog();
// 设置对话框的标题
openFileDialog.Title = "选择文件";
// 设置对话框的初始目录
openFileDialog.InitialDirectory = "C:\\";
// 设置对话框可以选择的文件类型,多个文件类型用分号隔开
openFileDialog.Filter = "文本文件|*.txt|所有文件|*.*";
// 显示对话框
if (openFileDialog.ShowDialog() == DialogResult.OK)
{
// 获取所选文件的路径
string filePath = openFileDialog.FileName;
Console.WriteLine("选择的文件路径:" + filePath);
}
```
在上述代码中,我们创建了一个 OpenFileDialog 对象,并设置了对话框的标题、初始目录和可以选择的文件类型。然后调用 ShowDialog 方法显示对话框,并判断用户是否选中了文件。如果用户选中了文件,则可以通过 FileName 属性获取所选文件的路径。
相关问题
C# response 以文件路径响应文件
你可以使用 ASP.NET Web API 来创建一个 Web 服务,读取指定路径的文件内容并将其作为文件下载响应返回给客户端。
以下是一个简单的示例代码:
```csharp
using System;
using System.IO;
using System.Net;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Web.Http;
public class FileController : ApiController
{
[HttpGet]
public HttpResponseMessage DownloadFile(string filePath)
{
try
{
// 检查文件是否存在
if (!File.Exists(filePath))
{
var response = new HttpResponseMessage(HttpStatusCode.NotFound);
response.Content = new StringContent("文件不存在:" + filePath);
return response;
}
// 读取文件内容
byte[] fileBytes = File.ReadAllBytes(filePath);
// 创建 HTTP 响应
var response = new HttpResponseMessage(HttpStatusCode.OK);
response.Content = new ByteArrayContent(fileBytes);
response.Content.Headers.ContentDisposition = new ContentDispositionHeaderValue("attachment");
response.Content.Headers.ContentDisposition.FileName = Path.GetFileName(filePath);
response.Content.Headers.ContentType = new MediaTypeHeaderValue("application/octet-stream");
return response;
}
catch (Exception e)
{
// 创建 HTTP 错误响应
var response = new HttpResponseMessage(HttpStatusCode.InternalServerError);
response.Content = new StringContent("读取文件失败:" + e.Message);
return response;
}
}
}
```
你需要在 Web 服务中注册该控制器,并启动 Web 服务。然后,你可以通过发送 GET 请求到 `/api/file?filePath=C:\example.txt` 来下载文件。如果文件读取成功,将返回 HTTP 200 OK 响应,并包含文件内容。如果文件不存在,将返回 HTTP 404 Not Found 响应,并包含错误消息。如果文件读取失败,将返回 HTTP 500 Internal Server Error 响应,并包含错误消息。另外,该代码还会设置响应头,以便浏览器能够正确地将文件下载到本地。
c#模糊查询本地文件
您好!对于在 C# 中进行模糊查询本地文件,您可以使用以下步骤:
1. 引入命名空间:首先,在您的代码文件中引入命名空间 `System.IO`。
2. 获取文件列表:使用 `Directory.GetFiles` 方法,传入要搜索的文件夹路径和搜索模式。搜索模式可以使用通配符 `*` 来表示模糊匹配。例如,如果要匹配所有以 `.txt` 结尾的文件,可以使用 `"*.txt"`。
3. 进行模糊查询:遍历获取到的文件列表,使用 `Path.GetFileName` 方法获取每个文件的文件名,并使用 `String.Contains` 方法来检查文件名是否包含您所需的模糊查询字符串。
下面是一个简单的示例代码,演示如何实现模糊查询本地文件:
```csharp
using System;
using System.IO;
class Program
{
static void Main()
{
string folderPath = "C:\\YourFolderPath"; // 替换为您要查询的文件夹路径
string searchPattern = "*.txt"; // 替换为您的模糊查询模式
string[] files = Directory.GetFiles(folderPath, searchPattern);
string query = "your query"; // 替换为您的模糊查询字符串
foreach (string filePath in files)
{
string fileName = Path.GetFileName(filePath);
if (fileName.Contains(query))
{
Console.WriteLine(filePath);
}
}
Console.ReadLine();
}
}
```
请确保替换示例代码中的文件夹路径、模糊查询模式和查询字符串,以适应您的实际需求。希望能对您有所帮助!如果您有任何疑问,请随时提问。
相关推荐
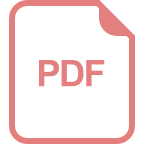
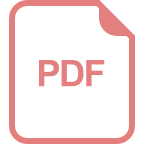












