如何用springboot 和RabbitMQ向指定用户发送通知
时间: 2024-03-09 10:20:59 浏览: 175
要用Spring Boot和RabbitMQ向指定用户发送通知,可以采用以下步骤:
1. 首先,需要在Spring Boot项目中集成RabbitMQ。可以在pom.xml中添加如下依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-amqp</artifactId>
</dependency>
```
2. 配置RabbitMQ连接信息,在application.yml中添加如下配置:
```
spring:
rabbitmq:
host: localhost
port: 5672
username: guest
password: guest
```
3. 创建一个消息实体类,用于向用户发送通知:
```java
public class NotificationMessage {
private String userId;
private String content;
// getter/setter 略
}
```
4. 创建一个消息发送者,用于将消息发送到RabbitMQ:
```java
@Component
public class NotificationSender {
private final RabbitTemplate rabbitTemplate;
public NotificationSender(RabbitTemplate rabbitTemplate) {
this.rabbitTemplate = rabbitTemplate;
}
public void send(NotificationMessage message) {
rabbitTemplate.convertAndSend("notification.exchange", "notification." + message.getUserId(), message);
}
}
```
5. 创建一个消息接收者,用于接收RabbitMQ中的消息并发送给对应的用户:
```java
@Component
public class NotificationReceiver {
private final SimpMessagingTemplate messagingTemplate;
public NotificationReceiver(SimpMessagingTemplate messagingTemplate) {
this.messagingTemplate = messagingTemplate;
}
@RabbitListener(queues = "notification.queue")
public void receive(NotificationMessage message) {
messagingTemplate.convertAndSendToUser(message.getUserId(), "/queue/notification", message.getContent());
}
}
```
6. 在控制器中调用消息发送者发送通知:
```java
@RestController
public class NotificationController {
private final NotificationSender sender;
public NotificationController(NotificationSender sender) {
this.sender = sender;
}
@PostMapping("/notification")
public void sendNotification(@RequestBody NotificationMessage message) {
sender.send(message);
}
}
```
这样,当调用/sendNotification接口时,就可以向指定用户发送通知了。其中,NotificationSender将消息发送到RabbitMQ的"notification.exchange"交换机,"notification." + message.getUserId()表示将消息发送到名为"notification.{userId}"的队列中;NotificationReceiver监听"notification.queue"队列,从中接收消息并发送给对应的用户。最后,在控制器中调用NotificationSender的send方法即可将消息发送到RabbitMQ。
阅读全文
相关推荐
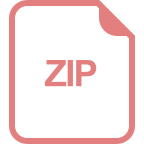



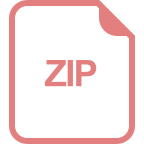
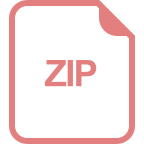
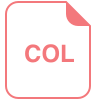
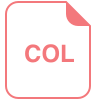



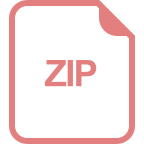
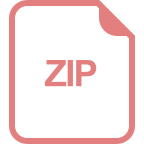
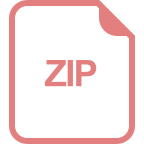