用微信小程序写一个登陆界面的代码
时间: 2023-09-27 17:05:55 浏览: 247
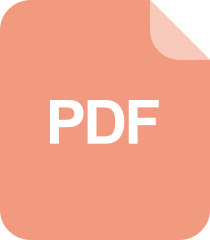
微信小程序登陆注册功能的实现代码

### 回答1:
当然可以,以下是一个简单的微信小程序登录界面的示例代码:
```
//index.js
Page({
data: {
username: '',
password: ''
},
// 监听输入框的输入事件
bindUsernameInput: function (e) {
this.setData({
username: e.detail.value
})
},
bindPasswordInput: function (e) {
this.setData({
password: e.detail.value
})
},
// 监听登录按钮的点击事件
bindLoginTap: function () {
// 在这里编写登录逻辑
// 可以向后端发送请求进行校验,也可以在前端进行简单的校验
if (this.data.username === 'admin' && this.data.password === '123456') {
wx.showToast({
title: '登录成功'
})
} else {
wx.showToast({
title: '用户名或密码错误',
icon: 'none'
})
}
}
})
```
```
<!--index.wxml-->
<view class="container">
<view class="input-box">
<text>用户名:</text>
<input bindinput="bindUsernameInput" value="{{username}}" placeholder="请输入用户名" />
</view>
<view class="input-box">
<text>密码:</text>
<input bindinput="bindPasswordInput" value="{{password}}" type="password" placeholder="请输入密码" />
</view>
<button class="login-btn" bindtap="bindLoginTap">登录</button>
</view>
```
```
/* index.wxss */
.container {
margin: 50rpx;
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
}
.input-box {
display: flex;
flex-direction: row;
align-items: center;
margin: 20rpx;
}
.text {
margin-right: 10rpx;
}
input {
border: 1rpx solid #ccc;
padding: 10rpx;
}
.login-btn {
width: 200rpx;
height: 40rpx;
background-color: #007aff;
color: #fff;
border-radius: 5rpx;
margin-top: 40rpx;
}
```
上面的代码实现了一个简单的微信小程序登录界面,包括输入框和登录按钮。在输入框中输入用户名和密码后,点击登录按钮即可进行登录操作。在实际的应用中,可以根据具体的需求进行修改和扩展。
### 回答2:
微信小程序是一种在微信中运行的小程序,可以用于开发各种功能性的小应用。下面是一个登陆界面的简单代码示例:
在小程序的.wxml文件中,添加如下代码:
```
<view class="container">
<view class="logo"></view>
<view class="input-container">
<input class="input" placeholder="请输入用户名" bindinput="bindUsernameInput" />
<input type="password" class="input" placeholder="请输入密码" bindinput="bindPasswordInput" />
</view>
<button class="login-btn" bindtap="login">登录</button>
</view>
```
在小程序的.wxss文件中,添加如下代码:
```
.container {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
height: 100vh;
}
.logo {
width: 100px;
height: 100px;
background-color: #ccc;
margin-bottom: 20px;
}
.input-container {
margin-bottom: 20px;
}
.input {
width: 300px;
height: 40px;
border: 1px solid #ccc;
border-radius: 5px;
padding: 5px;
margin-bottom: 10px;
}
.login-btn {
width: 300px;
height: 40px;
background-color: #333;
color: #fff;
border: none;
border-radius: 5px;
}
.login-btn:active {
background-color: #666;
}
```
在小程序的.js文件中,添加如下代码:
```
Page({
data: {
username: '',
password: ''
},
bindUsernameInput(e) {
this.setData({
username: e.detail.value
});
},
bindPasswordInput(e) {
this.setData({
password: e.detail.value
});
},
login() {
// 在这里编写登陆逻辑,比如调用接口进行验证
console.log('用户名:', this.data.username);
console.log('密码:', this.data.password);
}
})
```
以上代码实现了一个简单的登陆界面,包括用户名和密码的输入框以及一个登录按钮。当用户输入用户名和密码后,点击登录按钮会触发login方法,其中可以编写相关的登陆逻辑,比如调用接口进行验证。
### 回答3:
请注意,由于字数限制,无法提供完整的代码。以下是一个简单的微信小程序登陆界面的代码示例:
在wxml文件中:
```html
<view class="container">
<image class="logo" src="/images/logo.png"></image>
<text class="title">微信小程序登陆</text>
<input class="input" placeholder="请输入用户名" bindinput="bindUsernameInput"></input>
<input class="input" type="password" placeholder="请输入密码" bindinput="bindPasswordInput"></input>
<button class="button" bindtap="login">登陆</button>
</view>
```
在wxss文件中:
```css
.container {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
height: 100vh;
background-color: #fff;
}
.logo {
width: 80px;
height: 80px;
margin-bottom: 20px;
}
.title {
font-size: 22px;
font-weight: bold;
margin-bottom: 20px;
}
.input {
width: 300px;
height: 40px;
border: 1px solid #ccc;
border-radius: 5px;
padding: 0 10px;
margin-bottom: 10px;
}
.button {
width: 200px;
height: 40px;
background-color: #0084ff;
color: #fff;
border-radius: 5px;
}
```
在js文件中:
```javascript
Page({
data: {
username: '',
password: ''
},
bindUsernameInput: function(e) {
this.setData({
username: e.detail.value
});
},
bindPasswordInput: function(e) {
this.setData({
password: e.detail.value
});
},
login: function() {
// 在这里编写登陆逻辑
console.log('用户名:', this.data.username);
console.log('密码:', this.data.password);
}
});
```
这是一个简单的登陆界面,其中包含一个logo、一个标题、一个用户名输入框、一个密码输入框和一个登陆按钮。用户在输入用户名和密码后,点击登陆按钮可以触发登陆函数,你可以在该函数中编写具体的登陆逻辑。在示例中,登陆函数会将用户名和密码打印到控制台上。你可以根据实际需求在登陆函数中进行登陆操作,如发送请求给服务器验证用户信息。
阅读全文
相关推荐
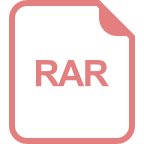




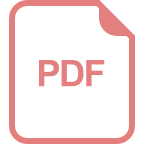
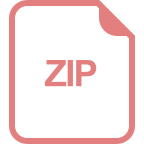
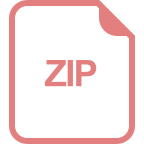
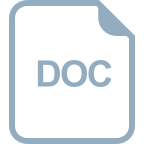


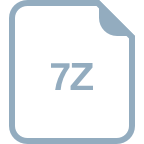
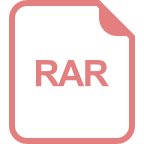