生产者-消费者问题(计算机系统中的许多问题都可归结为此问题)描述如下:生产者与消费者可以通过一个环形缓冲池联系起来,环形缓冲池由几个大小相等的缓冲块组成,每个缓冲块容纳一个产品。每个生产者可不断地每次往缓冲池中送一个生产产品,而每个消费者则可不断地每次从缓冲池中取出一个产品。 编写程序模拟多个生产者和多个消费者共享缓冲池的生产和消费过程。 要求: 1. 设计信号量用于进行生产者-消费者、生产者-生产者、消费者-消费者之间的同步控制; 2. 创建多个(不少于5个)进程或者线程模拟生产者和消费者; 3. 缓冲池大小定义在10-20,缓冲块中的产品为数据; 4. 程序运行结果能实时显示缓冲池中生产和消费产品的过程,包括断点、推进时间、次序等,编程语言可选用C、C++、Java或者Python。
时间: 2024-02-12 21:03:35 浏览: 20
非常感谢您的提问。根据您的问题,我可以为您提供以下回答:
1. 信号量是一种用于进程或线程同步的机制,可以实现生产者-消费者、生产者-生产者、消费者-消费者之间的同步控制。在这个问题中,您可以使用两个信号量:一个用于表示缓冲池中可用的缓冲块数量,另一个用于表示缓冲池中已满的缓冲块数量。当生产者生产一个缓冲块时,它会将空闲缓冲块数量信号量减1,同时将已满缓冲块数量信号量加1;当消费者消费一个缓冲块时,它会将空闲缓冲块数量信号量加1,同时将已满缓冲块数量信号量减1。
2. 为了模拟生产者和消费者的行为,您可以创建多个进程或线程。对于每个生产者进程或线程,它会不断地生产缓冲块并将其放入缓冲池中;对于每个消费者进程或线程,它会不断地从缓冲池中取出缓冲块并进行消费。需要注意的是,在多线程编程中,需要使用锁来保护共享资源的访问,以避免竞争条件和死锁等问题的出现。
3. 缓冲池大小可以定义为10-20,缓冲块中的产品可以是数据。具体来说,可以定义一个缓冲区数组,其大小为10-20。每个缓冲块可以包含一个数据项。当生产者生产缓冲块时,它会将数据项写入一个空闲的缓冲块中;当消费者消费缓冲块时,它会将缓冲块中的数据项读取出来并进行处理。
4. 程序运行结果可以实时显示缓冲池中生产和消费产品的过程,包括断点、推进时间、次序等。为了实现这一功能,您可以在程序中加入日志记录机制,记录每个生产者和消费者的行为,以及当前缓冲池中的状态。可以将日志输出到控制台或文件中,以便实时查看和分析。另外,在多线程编程中,需要注意线程的调度和同步问题,以确保程序的正确性和可靠性。
我推荐您使用Python编写此程序,因为Python有很多第三方库可以方便地实现多线程和日志记录等功能。您可以使用Python标准库中的threading模块来实现多线程,使用logging模块来实现日志记录。具体实现细节可以参考下面的代码示例。
代码示例:
```python
import threading
import time
import logging
BUFFER_SIZE = 10
BUFFER = [None] * BUFFER_SIZE
EMPTY_SEMAPHORE = threading.Semaphore(BUFFER_SIZE)
FULL_SEMAPHORE = threading.Semaphore(0)
LOCK = threading.Lock()
logging.basicConfig(level=logging.INFO)
class Producer(threading.Thread):
def __init__(self, id):
super().__init__()
self.id = id
def run(self):
global BUFFER
while True:
# Produce a new item
item = self.produce_item()
# Wait for an empty slot in the buffer
EMPTY_SEMAPHORE.acquire()
# Acquire the lock to protect the buffer
LOCK.acquire()
# Put the item in the buffer
index = self.get_next_empty_index()
BUFFER[index] = item
logging.info(f"Producer {self.id} produced item {item} at index {index}")
# Release the lock
LOCK.release()
# Signal that the buffer is no longer empty
FULL_SEMAPHORE.release()
# Sleep for a random amount of time
time.sleep(self.get_sleep_time())
def produce_item(self):
# TODO: Implement the item production logic here
return time.time()
def get_next_empty_index(self):
for i in range(BUFFER_SIZE):
if BUFFER[i] is None:
return i
return -1
def get_sleep_time(self):
return 1
class Consumer(threading.Thread):
def __init__(self, id):
super().__init__()
self.id = id
def run(self):
global BUFFER
while True:
# Wait for a full slot in the buffer
FULL_SEMAPHORE.acquire()
# Acquire the lock to protect the buffer
LOCK.acquire()
# Get the item from the buffer
index = self.get_next_full_index()
item = BUFFER[index]
BUFFER[index] = None
logging.info(f"Consumer {self.id} consumed item {item} at index {index}")
# Release the lock
LOCK.release()
# Signal that the buffer is no longer full
EMPTY_SEMAPHORE.release()
# Sleep for a random amount of time
time.sleep(self.get_sleep_time())
def get_next_full_index(self):
for i in range(BUFFER_SIZE):
if BUFFER[i] is not None:
return i
return -1
def get_sleep_time(self):
return 1
if __name__ == "__main__":
# Create multiple producers and consumers
producers = [Producer(i) for i in range(5)]
consumers = [Consumer(i) for i in range(5)]
# Start all the threads
for p in producers + consumers:
p.start()
# Wait for all the threads to finish
for p in producers + consumers:
p.join()
```
希望以上回答能够对您有所帮助。如果您有任何疑问或需要进一步的帮助,请随时告诉我。
相关推荐
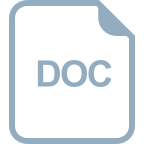
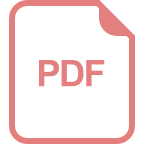
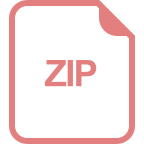



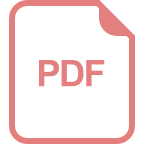
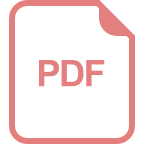
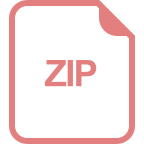
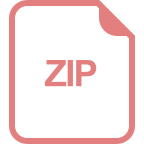
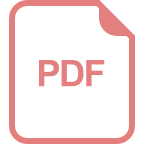
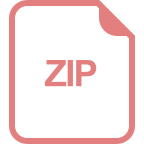
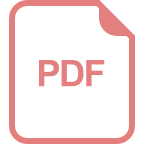
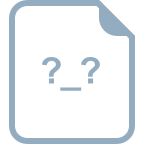
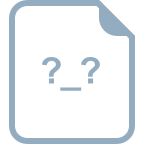