vue3 +typescript 登出之后重载页面
时间: 2023-08-10 11:39:47 浏览: 51
在Vue 3中,你可以使用`router.push()`方法来更新页面,以便在用户注销后将其重定向到登录页面。具体步骤如下:
1. 在你的`router/index.ts`文件中,导入`createRouter()`和`createWebHistory()`方法,然后使用它们来创建一个新的路由器实例。
```typescript
import { createRouter, createWebHistory } from 'vue-router'
const router = createRouter({
history: createWebHistory(),
routes: [...]
})
```
2. 在你的注销方法中,使用`router.push()`方法来将用户重定向到登录页面。
```typescript
import { useRouter } from 'vue-router'
export default {
setup() {
const router = useRouter()
const handleLogout = () => {
// 执行注销操作
router.push('/login')
}
return {
handleLogout
}
}
}
```
这将更新浏览器的URL,并将用户重定向到登录页面。如果你的登录页面是`/login`,你可以将上面的代码中的`/login`替换为你的登录页面的路由路径。
相关问题
Vue3 + TypeScript 项目经验
Vue3 + TypeScript 是一种常用的前端开发技术组合,它结合了Vue框架的便捷性和TypeScript的类型检查能力,可以提高项目的可维护性和开发效率。下面是一些Vue3 + TypeScript项目经验的介绍:
1. 项目初始化:使用Vue CLI创建一个Vue3项目,并选择TypeScript作为项目的语言。这样可以自动生成一些基本的配置和文件结构,方便快速开始开发。
2. 类型定义:在Vue3 + TypeScript项目中,可以使用TypeScript的类型系统来定义组件的props、data、computed等属性的类型,以及事件的参数类型等。这样可以在编码过程中及早发现潜在的类型错误,提高代码的健壮性。
3. 组件开发:在Vue3 + TypeScript项目中,可以使用装饰器(decorators)来定义组件选项,例如使用@Prop来定义props属性的类型,使用@Emit来定义事件的参数类型。这样可以更清晰地描述组件的接口和行为。
4. 路由管理:在Vue3 + TypeScript项目中,可以使用Vue Router进行路由管理。通过定义路由的类型和参数类型,可以在编码过程中获得更好的类型提示和错误检查。
5. 状态管理:在Vue3 + TypeScript项目中,可以使用Vuex进行状态管理。通过定义状态的类型和操作的参数类型,可以提高代码的可读性和可维护性。
6. 第三方库的使用:在Vue3 + TypeScript项目中,可以使用第三方库,并为其编写类型声明文件,以获得更好的类型检查和代码提示。例如,可以使用axios发送HTTP请求,并为其编写类型声明文件,以确保请求参数和响应数据的类型正确。
7. 单元测试:在Vue3 + TypeScript项目中,可以使用Jest等测试框架进行单元测试。通过编写类型安全的测试用例,可以提高代码的质量和可靠性。
8. 构建和部署:在Vue3 + TypeScript项目中,可以使用Webpack等构建工具进行项目的打包和优化。通过配置合适的TypeScript编译选项和Webpack插件,可以生成高效的生产环境代码。
vue3+typescript知识点
Vue3+TypeScript 是一种流行的前端开发组合。下面是一些你需要知道的 Vue3+TypeScript 的知识点:
1. 类型声明:TypeScript 为 Vue3 提供了类型声明文件,可以很方便地为 Vue3 应用程序添加类型支持。
2. 组件类型声明:TypeScript 为 Vue3 组件提供了类型声明,可以在组件中使用类型注释,以及使用泛型组件,从而提高代码的可读性和可维护性。
3. 装饰器:Vue3 支持装饰器语法,可以使用装饰器来简化组件的实现,并且可以在组件中使用装饰器来声明属性、方法和生命周期钩子等。
4. 模板类型检查:Vue3 的模板编译器支持模板类型检查,可以在编译期间检查模板中的类型错误,从而提高代码的可靠性和可维护性。
5. Composition API:Vue3 的 Composition API 提供了一种新的组件编写方式,可以更好地组织组件代码,并且可以使用 TypeScript 对 Composition API 进行类型检查。
6. 自定义指令类型声明:如果你在 Vue3 中使用了自定义指令,可以使用 TypeScript 为自定义指令添加类型声明,从而提高代码的可读性和可维护性。
7. 插件类型声明:如果你编写了 Vue3 插件,可以使用 TypeScript 为插件添加类型声明,从而提高代码的可读性和可维护性。
以上是 Vue3+TypeScript 的一些基本知识点,希望对你有所帮助。
相关推荐
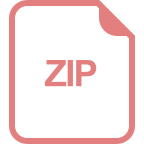
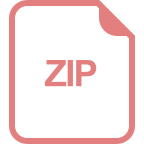
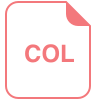
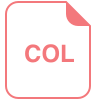
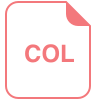
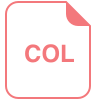
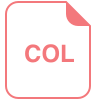







