用c 写hash table
时间: 2024-03-02 15:54:28 浏览: 17
好的,这里是用 C 语言实现的哈希表:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define SIZE 10
struct Node {
char* key;
int value;
struct Node* next;
};
struct Node* hash_table[SIZE];
int hash(char* key) {
int hash_value = 0;
int length = strlen(key);
for (int i = 0; i < length; i++) {
hash_value = hash_value + key[i];
}
return hash_value % SIZE;
}
void insert(char* key, int value) {
int index = hash(key);
struct Node* new_node = (struct Node*)malloc(sizeof(struct Node));
new_node->key = key;
new_node->value = value;
new_node->next = NULL;
if (hash_table[index] == NULL) {
hash_table[index] = new_node;
return;
}
struct Node* current_node = hash_table[index];
while (current_node->next != NULL) {
current_node = current_node->next;
}
current_node->next = new_node;
}
int get(char* key) {
int index = hash(key);
struct Node* current_node = hash_table[index];
while (current_node != NULL) {
if (strcmp(current_node->key, key) == 0) {
return current_node->value;
}
current_node = current_node->next;
}
return -1;
}
int main() {
insert("apple", 2);
insert("banana", 4);
insert("cherry", 6);
printf("Value of apple: %d\n", get("apple"));
printf("Value of banana: %d\n", get("banana"));
printf("Value of cherry: %d\n", get("cherry"));
return 0;
}
```
这个哈希表实现包括一个 `Node` 结构体来表示哈希表中的每个节点,它包含一个键值对和一个指向下一个节点的指针成员。`hash` 函数用于计算键的哈希值,`insert` 函数用于插入键值对,`get` 函数用于获取键对应的值。在 `main` 函数中,我们插入了几个键值对,然后使用 `get` 函数获取这些键对应的值,并打印出来。
相关推荐
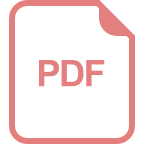














