qt使用tableview显示自定义数据结构
时间: 2023-10-24 19:21:17 浏览: 40
要在Qt中使用TableView显示自定义数据结构,你需要将该结构转换为Qt的模型(Model)。Qt的模型-视图(Model-View)框架提供了两种模型:标准模型和自定义模型。标准模型适用于表格和列表等简单的数据结构,而自定义模型则更加灵活,适用于显示自定义数据结构。
以下是一些步骤,可以帮助你在Qt中使用TableView显示自定义数据结构:
1. 创建自定义数据结构
首先,你需要创建一个自定义数据结构,例如一个包含姓名、年龄和性别的结构体。
```c++
struct Person {
QString name;
int age;
QString gender;
};
```
2. 创建自定义模型
接下来,你需要创建一个自定义模型,该模型将用于将自定义数据结构转换为Qt模型。你可以创建一个继承自QAbstractTableModel的类,并重写以下方法:
```c++
class PersonModel : public QAbstractTableModel
{
public:
PersonModel(QObject *parent = nullptr);
int rowCount(const QModelIndex &parent = QModelIndex()) const override;
int columnCount(const QModelIndex &parent = QModelIndex()) const override;
QVariant data(const QModelIndex &index, int role = Qt::DisplayRole) const override;
QVariant headerData(int section, Qt::Orientation orientation, int role = Qt::DisplayRole) const override;
QList<Person> persons;
private:
int _rowCount;
int _columnCount;
};
```
在上面的代码中,我们创建了一个名为PersonModel的自定义模型,并重写了rowCount、columnCount、data和headerData方法。
其中,rowCount和columnCount方法返回行数和列数,data方法返回单元格的数据,headerData方法返回表头的数据。
3. 实现自定义模型的方法
接下来,你需要实现自定义模型的方法。在这里,你需要将自定义数据结构转换为Qt模型的数据。在PersonModel类中,我们使用了一个QList来存储Person结构体的数据,因此我们需要在重写的方法中将该数据转换为Qt模型的数据。
```c++
int PersonModel::rowCount(const QModelIndex &parent) const
{
Q_UNUSED(parent);
return persons.count();
}
int PersonModel::columnCount(const QModelIndex &parent) const
{
Q_UNUSED(parent);
return 3;
}
QVariant PersonModel::data(const QModelIndex &index, int role) const
{
if (!index.isValid())
return QVariant();
if (index.row() >= persons.size() || index.row() < 0)
return QVariant();
if (role == Qt::DisplayRole || role == Qt::EditRole) {
const auto &person = persons.at(index.row());
if (index.column() == 0)
return person.name;
else if (index.column() == 1)
return person.age;
else if (index.column() == 2)
return person.gender;
}
return QVariant();
}
QVariant PersonModel::headerData(int section, Qt::Orientation orientation, int role) const
{
if (role != Qt::DisplayRole)
return QVariant();
if (orientation == Qt::Horizontal) {
switch (section) {
case 0:
return tr("Name");
case 1:
return tr("Age");
case 2:
return tr("Gender");
default:
return QVariant();
}
}
return QVariant();
}
```
在上面的代码中,我们重写了rowCount、columnCount、data和headerData方法,并将Person结构体的数据转换为Qt模型的数据。
4. 使用TableView显示自定义数据结构
最后,你可以使用TableView来显示自定义数据结构。在MainWindow类中,你可以创建一个TableView,并将它设置为PersonModel的视图。
```c++
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
, ui(new Ui::MainWindow)
{
ui->setupUi(this);
// create person model
PersonModel *personModel = new PersonModel(this);
personModel->persons.append({ "Tom", 25, "Male" });
personModel->persons.append({ "Alice", 30, "Female" });
personModel->persons.append({ "John", 40, "Male" });
// create table view
QTableView *tableView = new QTableView(this);
tableView->setModel(personModel);
// set table view properties
tableView->setSelectionMode(QAbstractItemView::SingleSelection);
tableView->setSelectionBehavior(QAbstractItemView::SelectRows);
tableView->setEditTriggers(QAbstractItemView::NoEditTriggers);
// set central widget
setCentralWidget(tableView);
}
```
在上面的代码中,我们创建了一个PersonModel,并将它设置为TableView的模型。然后,我们设置了TableView的一些属性,并将它设置为MainWindow的中央窗口部件。
这样,你就可以使用TableView来显示自定义数据结构了。
相关推荐
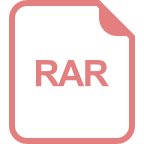










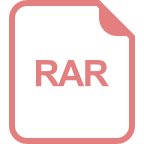