6.编写程序,程序中包含两个方法对命令行输入的两个整数进行运算。一个方法实现整数的整除运算,该方法捕获算数运算并进行处理;另一个方法实现两个整数的求余运算,该方法通过抛出异常的方式处理算数运算异常。
时间: 2023-06-11 21:05:11 浏览: 140
好的,您的第一个问题是编写程序,程序中包含两个方法对命令行输入的两个整数进行运算。一个方法实现整数的整除运算,该方法捕获算数运算并进行处理;另一个方法实现两个整数的求余运算,该方法通过抛出异常的方式处理算数运算异常。
以下是一个示例程序,可以满足您的需求:
```
import java.util.Scanner;
public class Arithmetic {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
int a, b;
System.out.print("Enter first number: ");
a = input.nextInt();
System.out.print("Enter second number: ");
b = input.nextInt();
try {
int quotient = divide(a, b);
System.out.println("Quotient: " + quotient);
} catch (ArithmeticException e) {
System.out.println(e.getMessage());
}
try {
int remainder = modulo(a, b);
System.out.println("Remainder: " + remainder);
} catch (ArithmeticException e) {
System.out.println(e.getMessage());
}
input.close();
}
public static int divide(int a, int b) throws ArithmeticException {
if (b == 0) {
throw new ArithmeticException("Cannot divide by zero!");
}
return a / b;
}
public static int modulo(int a, int b) throws ArithmeticException {
if (b == 0) {
throw new ArithmeticException("Cannot perform modulo operation with zero!");
}
return a % b;
}
}
```
该程序首先从命令行输入两个整数,然后调用 `divide()` 方法进行整除运算,并尝试捕获可能的算数异常。如果出现除以零的情况,该方法会抛出一个 `ArithmeticException` 异常,并在主程序中进行处理。
接下来,程序调用 `modulo()` 方法进行求余运算,同样尝试捕获算数异常。如果出现除以零的情况,该方法也会抛出一个 `ArithmeticException` 异常。
请注意,本程序只是一个示例,仅实现了简单的整除和求余运算。在实际应用中,还需要考虑更多的异常情况和错误处理。
阅读全文
相关推荐












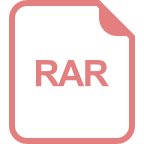



