Android 自定义FrameLayout,向右移动宽度减少
时间: 2024-05-06 09:15:57 浏览: 10
你可以通过重写 `onLayout()` 方法来实现自定义的 `FrameLayout`,并在其中实现向右移动宽度减少的效果。具体实现步骤如下:
1. 在你的 `FrameLayout` 类中重写 `onLayout()` 方法。
2. 在 `onLayout()` 方法中获取当前 `FrameLayout` 的宽度和高度。
3. 计算出需要向右移动的距离,并将子 View 对应的 left 和 right 坐标进行偏移。
4. 调用 `child.layout()` 方法重新布局子 View。
下面是一个示例代码,你可以参考一下:
```java
public class CustomFrameLayout extends FrameLayout {
public CustomFrameLayout(Context context) {
super(context);
}
public CustomFrameLayout(Context context, AttributeSet attrs) {
super(context, attrs);
}
public CustomFrameLayout(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
}
@Override
protected void onLayout(boolean changed, int left, int top, int right, int bottom) {
super.onLayout(changed, left, top, right, bottom);
// 获取当前 FrameLayout 的宽度和高度
int width = getWidth();
int height = getHeight();
// 需要向右移动的距离
int offsetX = width / 2;
// 遍历所有子 View
for (int i = 0; i < getChildCount(); i++) {
View child = getChildAt(i);
// 获取子 View 的 left 和 right 坐标
int childLeft = child.getLeft();
int childRight = child.getRight();
// 向右移动宽度减少的距离
childLeft += offsetX;
childRight -= offsetX;
// 重新布局子 View
child.layout(childLeft, child.getTop(), childRight, child.getBottom());
}
}
}
```
如果你使用了 Kotlin,可以参考以下代码:
```kotlin
class CustomFrameLayout @JvmOverloads constructor(
context: Context,
attrs: AttributeSet? = null,
defStyleAttr: Int = 0
) : FrameLayout(context, attrs, defStyleAttr) {
override fun onLayout(changed: Boolean, left: Int, top: Int, right: Int, bottom: Int) {
super.onLayout(changed, left, top, right, bottom)
// 获取当前 FrameLayout 的宽度和高度
val width = width
val height = height
// 需要向右移动的距离
val offsetX = width / 2
// 遍历所有子 View
for (i in 0 until childCount) {
val child = getChildAt(i)
// 获取子 View 的 left 和 right 坐标
var childLeft = child.left
var childRight = child.right
// 向右移动宽度减少的距离
childLeft += offsetX
childRight -= offsetX
// 重新布局子 View
child.layout(childLeft, child.top, childRight, child.bottom)
}
}
}
```
这样,你就可以在布局文件中使用自定义的 `CustomFrameLayout`,并在其中实现向右移动宽度减少的效果了。
相关推荐
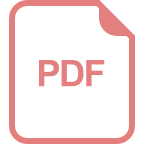
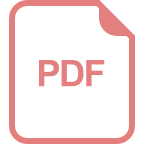
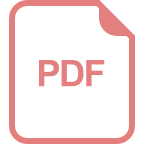














