在Java中以"年-月-日 小时:分钟:秒"的格式显示当前时间,年4位,月、日、小时、分钟、秒占2位,小时是24进制的。
时间: 2023-09-05 07:04:56 浏览: 94
### 回答1:
可以使用SimpleDateFormat类来实现这个功能。代码示例如下:
```
import java.text.SimpleDateFormat;
import java.util.Date;
public class Main {
public static void main(String[] args) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
String currentTime = sdf.format(new Date());
System.out.println(currentTime);
}
}
```
输出结果为当前时间的字符串,格式为:年-月-日 小时:分钟:秒。
### 回答2:
在Java中,可以使用SimpleDateFormat类来以指定的日期时间格式显示当前时间。具体代码如下:
```java
import java.text.SimpleDateFormat;
import java.util.Date;
public class Main {
public static void main(String[] args) {
SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
Date date = new Date();
String formattedDate = formatter.format(date);
System.out.println("当前时间: " + formattedDate);
}
}
```
这段代码首先创建了一个SimpleDateFormat对象,并指定日期时间格式为"yyyy-MM-dd HH:mm:ss"。然后,使用Date类获取当前时间,并通过SimpleDateFormat的format()方法将其格式化成指定格式的字符串。最后,通过System.out.println()方法将格式化后的时间字符串输出到控制台。
例如,如果当前时间是2022年5月1日14点30分25秒,那么输出结果将是"当前时间: 2022-05-01 14:30:25"。其中,年份占4位,月、日、小时、分钟、秒分别占2位。小时使用24小时制显示。
请注意,由于Date类和SimpleDateFormat类都位于java.util包下,因此在使用该代码时需要进行相应的导入。
### 回答3:
在Java中,可以通过使用SimpleDateFormat类来格式化当前时间并以"年-月-日 小时:分钟:秒"的形式显示。具体步骤如下:
1. 导入SimpleDateFormat类:
```java
import java.text.SimpleDateFormat;
```
2. 创建SimpleDateFormat对象,指定日期时间的格式:
```java
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
```
3. 获取当前时间:
```java
java.util.Date date = new java.util.Date();
```
4. 使用SimpleDateFormat对象将当前时间格式化为字符串:
```java
String formattedDate = sdf.format(date);
```
这样,formattedDate变量中的值就是当前时间按照"年-月-日 小时:分钟:秒"格式的字符串。
完整的代码如下:
```java
import java.text.SimpleDateFormat;
public class Main {
public static void main(String[] args) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
java.util.Date date = new java.util.Date();
String formattedDate = sdf.format(date);
System.out.println(formattedDate);
}
}
```
运行该代码,将会以"年-月-日 小时:分钟:秒"的格式显示当前的日期时间。
相关推荐
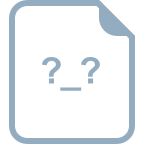
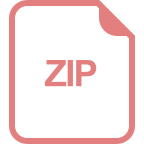














