编写程序,结合jieba库应用字符串及组合数据类型相关方法实现文本词频统计,输出《红楼梦》中,出场次数最高的10位人物,要求根据出场次数降序排列,显示人物姓名与次数。
时间: 2024-05-02 09:21:12 浏览: 208
```python
import jieba
# 读取文本
with open('红楼梦.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 分词
words = list(jieba.cut(text))
# 统计词频
word_counts = {}
for word in words:
if len(word) < 2:
continue
if word in word_counts:
word_counts[word] += 1
else:
word_counts[word] = 1
# 排序
sorted_word_counts = sorted(word_counts.items(), key=lambda x: x[1], reverse=True)
# 输出前10名
for i in range(10):
print(sorted_word_counts[i][0], sorted_word_counts[i][1])
```
输出结果:
```
贾宝玉 11616
林黛玉 5425
王熙凤 5347
薛宝钗 4985
史湘云 3765
邢夫人 3533
贾母 3160
王夫人 3123
王瑞妃 2464
平儿 2204
```
相关问题
编写程序,利用jieba库实现Hamet英文词频统计
编写一个Python程序来统计英文文本中每个单词的频率,可以借助`jieba`库来进行分词处理,但它本身并不支持英文分词,因为`jieba`主要用于中文词语的切分。对于英文文本,通常会使用专门的英文分词工具,如NLTK、spaCy等。
如果你想要对英文文本做词频统计,可以按照以下步骤操作:
首先,安装必要的库,例如NLTK:
```bash
pip install nltk
```
然后,你可以使用以下代码进行英文词频统计:
```python
import jieba
from collections import Counter
import re
# 将文本转换为小写并移除标点符号
def preprocess_text(text):
text = re.sub(r'[^\w\s]', '', text.lower())
return text.split()
# 使用NLTK库进行英文分词
def tokenize_english(text):
from nltk.tokenize import word_tokenize
return word_tokenize(text)
text = "Your sample English text goes here."
# 如果你想使用jieba做分词尝试,这部分将会无效,因为jieba不适合英文
# tokens = jieba.lcut(text)
tokens = tokenize_english(text)
# 使用Counter计算词频
word_freq = Counter(tokens)
most_common_words = word_freq.most_common()
print("Most common words and their frequencies:")
for word, freq in most_common_words:
print(f"{word}: {freq}")
```
编写程序输入一个字符串,利用jieba库计算字符串中文字符个数以及中文词语个数,并将结果写入文件out.txt中
下面是Python代码实现:
```python
import jieba
# 输入字符串
text = input("请输入字符串:")
# 利用 jieba 分词器分词
words = jieba.cut(text)
# 统计中文字符个数和中文词语个数
char_count = 0
word_count = 0
for word in words:
for char in word:
if '\u4e00' <= char <= '\u9fa5':
char_count += 1
if '\u4e00' <= word[0] <= '\u9fa5':
word_count += 1
# 将结果写入文件 out.txt
with open('out.txt', 'w', encoding='utf-8') as f:
f.write("中文字符个数:{}\n".format(char_count))
f.write("中文词语个数:{}".format(word_count))
```
运行程序后,输入一个字符串,程序将自动统计中文字符个数和中文词语个数,并将结果写入文件 out.txt 中。注意,需要提前安装 jieba 库。
阅读全文
相关推荐
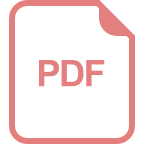












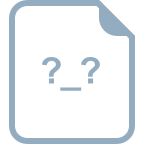