使用Selenium前,如何导入证书到浏览器?
时间: 2023-12-29 13:02:36 浏览: 33
要导入证书到浏览器,需要执行以下步骤:
1. 在浏览器中打开网站,该网站需要使用证书。
2. 在浏览器地址栏中点击锁形状的图标,打开安全信息面板。
3. 点击“证书”选项卡,然后选择“查看证书”按钮。
4. 在弹出的证书窗口中,选择“详细信息”选项卡。
5. 在“详细信息”选项卡中,找到“导出”按钮,选择导出证书。
6. 在导出证书窗口中,选择证书格式(例如,以.pfx或.cer形式导出)并设置密码。
7. 将证书文件保存到本地计算机上的某个位置。
接下来,您可以使用Selenium WebDriver将证书导入浏览器:
1. 在WebDriver启动浏览器之前,将以下代码添加到程序中:
```python
from selenium.webdriver.chrome.options import Options
chrome_options = Options()
chrome_options.add_argument('--ignore-certificate-errors')
chrome_options.add_argument('--allow-running-insecure-content')
```
这将忽略证书错误并允许加载不安全内容。
2. 启动WebDriver时,将以下代码添加到程序中:
```python
from selenium import webdriver
driver = webdriver.Chrome(chrome_options=chrome_options)
```
这将在启动Chrome浏览器时使用上面设置的选项。
3. 使用以下代码导入证书:
```python
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.chrome.options import Options
from selenium import webdriver
chrome_options = Options()
chrome_options.add_argument('--ignore-certificate-errors')
chrome_options.add_argument('--allow-running-insecure-content')
service = Service('/path/to/chromedriver')
service.start()
driver = webdriver.Remote(service.service_url, desired_capabilities=chrome_options.to_capabilities())
# 将证书导入浏览器
cert_file_path = '/path/to/cert/file'
driver.get('chrome://settings/certificates')
driver.find_element_by_xpath("//span[text()='Authorities']").click()
driver.find_element_by_xpath("//span[text()='Import...']").click()
driver.find_element_by_css_selector("input[type='file']").send_keys(cert_file_path)
driver.find_element_by_xpath("//span[text()='Open']").click()
driver.find_element_by_xpath("//span[text()='Done']").click()
# 关闭浏览器
driver.quit()
```
在上面的代码中,我们使用Selenium WebDriver打开Chrome浏览器并导入证书。请注意,需要将`/path/to/chromedriver`替换为您计算机上Chrome驱动程序的实际路径。同样,需要将`/path/to/cert/file`替换为您的证书文件的实际路径。
相关推荐
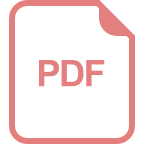
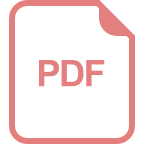
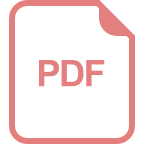














