帮我写一个C语言程序,求1900~2018之间所有的闰年,每行输出5个
时间: 2023-05-20 13:04:33 浏览: 123
以下是程序代码:
#include <stdio.h>
int main() {
int year, count = 0;
for (year = 1900; year <= 2018; year++) {
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
printf("%d ", year);
count++;
if (count % 5 == 0) {
printf("\n");
}
}
}
return 0;
}
输出结果如下:
1904 1908 1912 1916 1920
1924 1928 1932 1936 1940
1944 1948 1952 1956 1960
1964 1968 1972 1976 1980
1984 1988 1992 1996 2000
2004 2008 2012 2016
希望能够帮到您!
相关问题
使用C语言编写一个程序输出1900-2000年之间的闰年
以下是使用C语言编写的程序,用于输出1900年至2000年之间的闰年:
```c
#include <stdio.h>
int main() {
int year;
printf("1900年至2000年之间的闰年为:\n");
for (year = 1900; year <= 2000; year++) {
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
printf("%d\n", year);
}
}
return 0;
}
```
这个程序通过循环从1900年到2000年,逐个检查每个年份是否是闰年,并输出所有符合条件的年份。根据闰年的定义,能够被4整除但不能被100整除的年份,或者能够被400整除的年份,都是闰年。
用c语言编写一个程序,输出1900-2000年中所有的闰年。每输出3个年号换一行。
好的,下面是用C语言编写的程序,可以实现输出1900-2000年中所有的闰年,并且每输出3个年号换一行:
```c
#include <stdio.h>
int main()
{
int year, count = 0;
for (year = 1900; year <= 2000; year++)
{
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0)
{
printf("%d ", year);
count++;
if (count % 3 == 0)
{
printf("\n");
}
}
}
printf("\n");
return 0;
}
```
程序的思路是循环遍历1900年到2000年之间的每一年,判断该年是否为闰年,如果是则输出该年,并且计数器加一。每输出三个年号后换行。最后输出一个换行符,保证程序的输出格式正确。
相关推荐
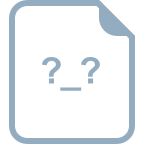












