从https://blog.csdn.net/ZhouYaNan1029/article/details/80401253上将各国的信息(英文名称、中文名称、昵称等)爬取下来, 并以json格式存放到本地用lxml库
时间: 2023-08-08 08:05:18 浏览: 99
### 回答1:
可以使用 Python 的 requests 和 lxml 库来实现这个功能。以下是一个实现的示例代码:
```python
import requests
from lxml import etree
import json
url = 'https://blog.csdn.net/ZhouYaNan1029/article/details/80401253'
response = requests.get(url)
html = response.content.decode('utf-8')
selector = etree.HTML(html)
# 获取表格中的数据
table = selector.xpath('//table')[0]
rows = table.xpath('.//tr')
data = []
for row in rows:
cols = row.xpath('.//td')
if len(cols) == 3:
country = {}
country['english_name'] = cols[0].text.strip()
country['chinese_name'] = cols[1].text.strip()
country['nickname'] = cols[2].text.strip()
data.append(country)
# 将数据以 JSON 格式保存到本地
with open('countries.json', 'w', encoding='utf-8') as f:
json.dump(data, f, ensure_ascii=False, indent=4)
```
这个程序会将 https://blog.csdn.net/ZhouYaNan1029/article/details/80401253 页面上的表格数据爬取下来,并以 JSON 格式保存到本地文件 countries.json 中。
注意:在运行代码前需要安装 requests 和 lxml 库,可以使用 pip 进行安装。
### 回答2:
首先,我们将使用Python的requests库来获取网页内容。然后,我们使用lxml库来解析HTML并提取所需的信息。最后,将提取到的信息转换为JSON格式并存储到本地文件中。
下面是具体的步骤:
1. 导入所需的库:
```python
import requests
from lxml import etree
import json
```
2. 使用requests库来获取网页内容:
```python
url = 'https://blog.csdn.net/ZhouYaNan1029/article/details/80401253'
response = requests.get(url)
html = response.text
```
3. 使用lxml库解析HTML:
```python
tree = etree.HTML(html)
```
4. 使用XPath定位并提取需要的元素:
```python
countries = tree.xpath('//table//tr')[1:] # 跳过表头
result = {}
for country in countries:
en_name = country.xpath('./td[1]//text()')[0]
cn_name = country.xpath('./td[2]//text()')[0]
nickname = country.xpath('./td[3]//text()')[0]
result[en_name] = {
'中文名称': cn_name,
'昵称': nickname
}
```
5. 将提取到的信息转换为JSON格式并存储到本地文件:
```python
json_data = json.dumps(result, ensure_ascii=False)
with open('countries.json', 'w', encoding='utf-8') as f:
f.write(json_data)
```
最终,将各国的信息以JSON格式存储到了名为 "countries.json" 的文件中。
请注意,此代码可能需要根据链接中网页的实际结构进行微调。另外,必要时,您可能需要安装相关的库。
### 回答3:
爬取各国的信息可以通过使用Python的requests库来发送请求,通过lxml库来解析网页内容,并使用json库将数据以json格式存放到本地。具体步骤如下:
1. 导入所需库:
```python
import requests
from lxml import etree
import json
```
2. 发送请求并获取网页内容:
```python
url = "https://blog.csdn.net/ZhouYaNan1029/article/details/80401253"
response = requests.get(url)
html = response.content
```
3. 使用lxml库解析网页内容并获取所需信息:
```python
tree = etree.HTML(html)
country_list = tree.xpath('//table//tr[position()>1]')
```
4. 遍历解析出的信息,提取各国的英文名称、中文名称、昵称等,并存放到一个字典列表中:
```python
result = []
for country in country_list:
info = {}
info['english_name'] = country.xpath('./td[1]/text()')[0]
info['chinese_name'] = country.xpath('./td[2]/text()')[0]
info['nickname'] = country.xpath('./td[3]/text()')[0]
result.append(info)
```
5. 将数据以json格式存放到本地文件:
```python
with open('countries.json', 'w', encoding='utf-8') as f:
json.dump(result, f, ensure_ascii=False)
```
以上是使用Python进行爬取各国信息,并以json格式存放到本地的简单实现。注意,实际应用中还需要进行异常处理、数据清洗等相关操作。
阅读全文
相关推荐
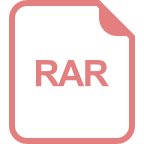
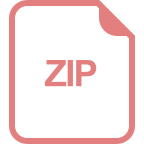
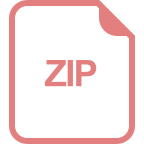















