获取painter->drawArc绘制完成后弧线边缘的中心坐标
时间: 2023-11-28 21:49:55 浏览: 152
要获取绘制完成后弧线边缘的中心坐标,可以通过以下步骤实现:
1. 使用QPainterPath构建弧线路径,设置起始角度和结束角度,并使用QPainterPathStroker指定线条宽度和线条样式。
2. 使用QPainter将路径绘制到QPixmap上。
3. 使用QPixmap.toImage()将QPixmap转换为QImage对象。
4. 遍历QImage像素,找到弧线边缘的像素点。
5. 计算这些像素点的中心坐标。
下面是一个示例代码,演示了如何获取绘制完成后弧线边缘的中心坐标:
```python
import sys
from PyQt5.QtCore import Qt
from PyQt5.QtGui import QPainter, QPainterPath, QPixmap, QImage, QColor, QPen
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QHBoxLayout, QVBoxLayout
class Example(QWidget):
def __init__(self):
super().__init__()
hbox = QHBoxLayout()
pixmap = QPixmap(400, 400)
pixmap.fill(Qt.white)
painter = QPainter(pixmap)
path = QPainterPath()
path.arcTo(100, 100, 200, 200, 45, 90)
stroker = QPen()
stroker.setWidth(10)
stroker.setStyle(Qt.SolidLine)
stroker.setCapStyle(Qt.FlatCap)
stroker.setJoinStyle(Qt.MiterJoin)
painter.strokePath(path, stroker)
painter.end()
image = pixmap.toImage()
center_points = []
for y in range(image.height()):
for x in range(image.width()):
color = QColor(image.pixel(x, y))
if color == QColor(Qt.black):
center_points.append((x, y))
print(center_points)
hbox.addWidget(QLabel(pixmap=pixmap))
self.setLayout(hbox)
self.setGeometry(300, 300, 500, 500)
self.setWindowTitle('Example')
self.show()
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = Example()
sys.exit(app.exec_())
```
在这个示例中,我们首先创建一个400x400的QPixmap,并使用QPainter绘制一个起始角度为45度、结束角度为135度的圆弧。我们将线条宽度设置为10,并使用Qt.SolidLine样式绘制线条。
接下来,我们使用QPixmap.toImage()将QPixmap转换为QImage对象,并遍历QImage像素,找到所有的黑色像素点。我们假设这些黑色像素点就是弧线边缘的像素点,并计算它们的中心坐标。
在这个示例中,我们只是简单地打印出了所有的中心点坐标。如果你想在图形界面上显示这些点,可以将它们绘制出来,例如使用QPainter在QPixmap上绘制小圆点。
阅读全文
相关推荐
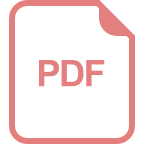



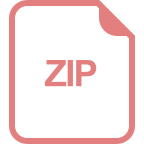
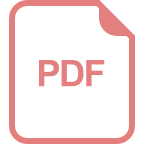

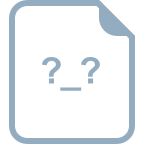
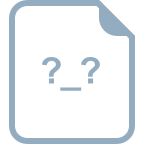