用socketAPI实现一个文件管理系统
时间: 2024-05-07 12:19:02 浏览: 44
一个简单的文件管理系统可以通过socket API实现,以下是一个基础的Python实现:
```python
import socket
import os
HOST = '127.0.0.1'
PORT = 8888
def list_files(conn):
files = os.listdir('.')
files_str = ""
for file in files:
files_str += file + "\n"
conn.sendall(files_str.encode())
def download_file(conn, filename):
if os.path.isfile(filename):
conn.sendall("EXIST".encode())
user_response = conn.recv(1024).decode()
if user_response[:2] == 'OK':
with open(filename, 'rb') as f:
bytes_to_send = f.read(1024)
conn.sendall(bytes_to_send)
while bytes_to_send != "":
bytes_to_send = f.read(1024)
conn.sendall(bytes_to_send)
else:
conn.sendall("ERROR".encode())
conn.close()
def upload_file(conn, filename):
if os.path.isfile(filename):
conn.sendall("EXIST".encode())
return
else:
conn.sendall("OK".encode())
with open(filename, 'wb') as f:
while True:
data = conn.recv(1024)
if not data:
break
f.write(data)
conn.close()
def main():
s = socket.socket()
s.bind((HOST, PORT))
s.listen(5)
print("Server started...")
while True:
conn, addr = s.accept()
print("Client connected: ", addr)
user_choice = conn.recv(1024).decode()
if user_choice == "1":
list_files(conn)
elif user_choice == "2":
filename = conn.recv(1024).decode()
download_file(conn, filename)
elif user_choice == "3":
filename = conn.recv(1024).decode()
upload_file(conn, filename)
else:
conn.sendall("INVALID".encode())
print("Client disconnected: ", addr)
s.close()
if __name__ == '__main__':
main()
```
该代码实现了三种基本操作:列出文件列表、上传文件和下载文件。在主函数中,服务器侦听客户端连接并接收客户端的选项,然后根据选项执行相应的操作。对于上传和下载文件,客户端需要提供文件名并进行相应的确认操作。
阅读全文
相关推荐

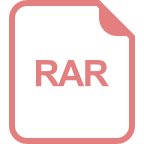
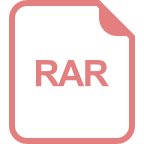
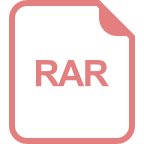
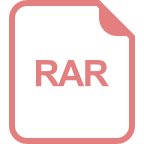



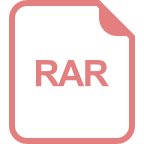


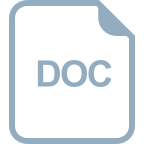



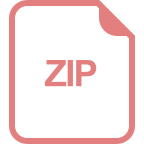
