如何让IOS富文本支持background: linear-gradient(90deg, black 50%, #D3D3D3 50%);-webkit-background-clip: text;-webkit-text-fill-color: transparent;
时间: 2023-09-06 22:07:48 浏览: 183
要让iOS富文本支持该样式,你可以使用Core Text框架来实现。下面是一个示例代码:
```swift
import UIKit
import CoreText
extension NSMutableAttributedString {
func applyLinearGradient(colors: [CGColor], startPoint: CGPoint, endPoint: CGPoint) {
let gradient = CGGradient(colorsSpace: CGColorSpaceCreateDeviceRGB(), colors: colors as CFArray, locations: nil)!
let stringRange = NSRange(location: 0, length: self.length)
let line = CTLineCreateWithAttributedString(self)
let runs = CTLineGetGlyphRuns(line)
for runIndex in 0..<CFArrayGetCount(runs) {
let run = CFArrayGetValueAtIndex(runs, runIndex)!
let runRange = CTRunGetStringRange(run)
var runRect = CGRect.zero
CTRunGetTypographicBounds(run, CFRangeMake(0, 0), &runRect.origin, &runRect.size, nil)
let glyphCount = CTRunGetGlyphCount(run)
var glyphPositions = Array<CGPoint>(repeating: .zero, count: glyphCount)
CTRunGetPositions(run, CFRangeMake(0, 0), &glyphPositions)
for glyphIndex in 0..<glyphCount {
let glyphRange = CFRangeMake(glyphIndex, 1)
let position = glyphPositions[glyphIndex]
let glyphRect = CGRect(x: runRect.origin.x + position.x, y: runRect.origin.y + position.y, width: runRect.size.width, height: runRect.size.height)
let glyphLocation = runRange.location + glyphRange.location
let characterRange = CFRangeMake(glyphLocation, glyphRange.length)
let tokenRange = CTLineGetStringRange(line)
let adjustedRange = CFRangeMake(characterRange.location - tokenRange.location, characterRange.length)
self.enumerateAttribute(.font, in: adjustedRange, options: []) { (value, range, stop) in
let font = value as! UIFont
let fontSize = font.pointSize
let glyphPath = CTFontCreatePathForGlyph(font, glyphRange.location, nil)!
let glyphBoundingBox = glyphPath.boundingBox
let textMatrix = CGAffineTransform(scaleX: 1, y: -1).translatedBy(x: 0, y: -runRect.size.height)
let glyphTransform = glyphBoundingBox.applying(textMatrix)
let startPoint = CGPoint(x: startPoint.x + glyphRect.origin.x, y: startPoint.y + glyphRect.origin.y)
let endPoint = CGPoint(x: endPoint.x + glyphRect.origin.x, y: endPoint.y + glyphRect.origin.y)
let gradientTransform = CGAffineTransform(translationX: startPoint.x, y: startPoint.y).scaledBy(x: (endPoint.x - startPoint.x) / glyphBoundingBox.width, y: (endPoint.y - startPoint.y) / glyphBoundingBox.height)
let gradientPath = glyphPath.copy(using: &gradientTransform)!
let gradientBoundingBox = gradientPath.boundingBox
let boundingBoxTransform = CGAffineTransform(translationX: -gradientBoundingBox.origin.x, y: -gradientBoundingBox.origin.y)
let transformedGradientPath = gradientPath.copy(using: &boundingBoxTransform)!
let context = UIGraphicsGetCurrentContext()!
context.addPath(transformedGradientPath)
context.clip()
context.drawLinearGradient(gradient, start: startPoint, end: endPoint, options: [.drawsBeforeStartLocation, .drawsAfterEndLocation])
}
}
}
}
}
// 使用示例
let label = UILabel()
let attributedText = NSMutableAttributedString(string: "Your text here")
let colors = [UIColor.black.cgColor, UIColor(red: 211/255, green: 211/255, blue: 211/255, alpha: 1).cgColor]
let startPoint = CGPoint(x: 0, y: 0)
let endPoint = CGPoint(x: label.bounds.width, y: 0)
attributedText.addAttribute(.foregroundColor, value: UIColor.clear, range: NSRange(location: 0, length: attributedText.length))
label.attributedText = attributedText
label.applyLinearGradient(colors: colors, startPoint: startPoint, endPoint: endPoint)
```
通过上述代码,你可以将UILabel的文本设置为富文本,并应用线性渐变背景效果。请注意,这个代码示例是使用Swift编写的,所以你需要在你的iOS项目中创建一个适当的代码文件,并将其添加到你的项目中。
阅读全文
相关推荐
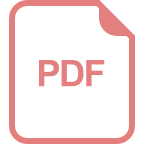
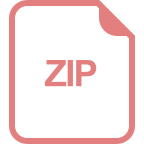

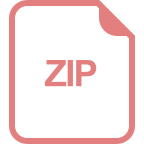
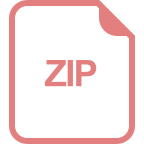
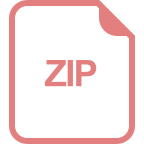
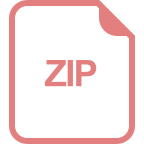
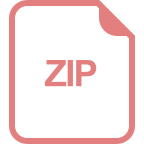
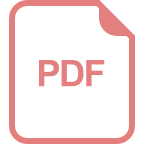
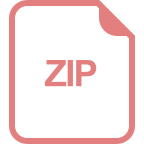
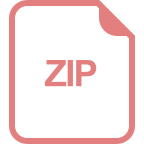
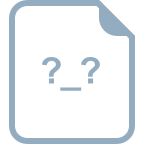
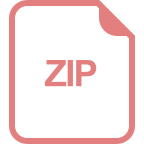
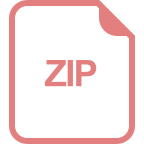
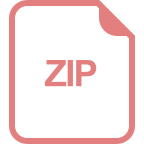
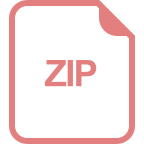
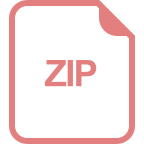
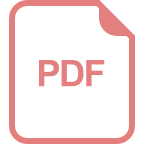