qt如何使用Linux内核的PCIe驱动程序库
时间: 2024-05-09 07:21:23 浏览: 10
在Qt中使用Linux内核的PCIe驱动程序库需要以下步骤:
1. 安装Linux内核开发工具包(kernel-devel)和Qt开发工具包(Qt-devel)。
2. 编写PCIe驱动程序库的代码并编译生成.so动态链接库文件。
3. 在Qt项目中添加PCIe驱动程序库的头文件路径和.so文件路径。
4. 在Qt项目中使用PCIe驱动程序库的API函数。
具体操作步骤如下:
1. 安装Linux内核开发工具包和Qt开发工具包:
```bash
sudo apt-get install kernel-devel
sudo apt-get install qt5-devel
```
2. 编写PCIe驱动程序库的代码并编译生成.so动态链接库文件:
```c
#include <linux/module.h>
#include <linux/pci.h>
int my_pci_probe(struct pci_dev *pdev, const struct pci_device_id *id)
{
// TODO: PCIe驱动程序库的代码
return 0;
}
void my_pci_remove(struct pci_dev *pdev)
{
// TODO: PCIe驱动程序库的代码
}
static struct pci_device_id my_pci_tbl[] = {
{ PCI_DEVICE(0x8086, 0x100e), },
{ 0, }
};
MODULE_DEVICE_TABLE(pci, my_pci_tbl);
static struct pci_driver my_pci_driver = {
.name = "my_pci_driver",
.id_table = my_pci_tbl,
.probe = my_pci_probe,
.remove = my_pci_remove,
};
static int __init my_pci_init(void)
{
return pci_register_driver(&my_pci_driver);
}
static void __exit my_pci_exit(void)
{
pci_unregister_driver(&my_pci_driver);
}
module_init(my_pci_init);
module_exit(my_pci_exit);
MODULE_AUTHOR("Your Name");
MODULE_DESCRIPTION("PCIe driver library");
MODULE_LICENSE("GPL");
```
编译生成.so动态链接库文件:
```bash
make -C /lib/modules/$(uname -r)/build M=$(pwd) modules
```
3. 在Qt项目中添加PCIe驱动程序库的头文件路径和.so文件路径:
在Qt项目的.pro文件中添加以下内容:
```qmake
INCLUDEPATH += /usr/src/linux-headers-$(uname -r)/include
LIBS += -L/path/to/pci_driver_library -lpci_driver_library
```
4. 在Qt项目中使用PCIe驱动程序库的API函数:
```c++
#include <pci/pci.h>
pci_access *pacc;
pacc = pci_alloc();
pci_init(pacc);
pci_scan_bus(pacc);
for (struct pci_dev *pdev = pacc->devices; pdev; pdev = pdev->next) {
// TODO: 使用PCIe驱动程序库的API函数
}
pci_cleanup(pacc);
```
相关推荐
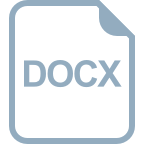
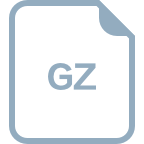














