c++不用第三方库将string转化成xml
时间: 2023-07-26 08:22:17 浏览: 146
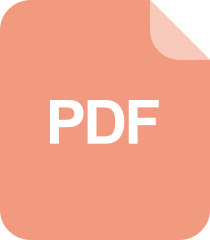
常用C++第三方库
在C++中,可以使用标准库中的sstream和tinyxml2库来将一个字符串转换为XML格式。
具体步骤如下:
1. 引入头文件
```cpp
#include <iostream>
#include <sstream>
#include "tinyxml2.h"
```
2. 创建一个XML文档
```cpp
tinyxml2::XMLDocument doc;
```
3. 创建一个根元素
```cpp
tinyxml2::XMLElement* root = doc.NewElement("root");
doc.InsertEndChild(root);
```
4. 将字符串解析为XML格式
```cpp
std::istringstream iss(str);
tinyxml2::XMLDocument tempDoc;
tempDoc.Parse(str.c_str());
tinyxml2::XMLElement* node = tempDoc.FirstChildElement();
while(node) {
tinyxml2::XMLElement* copy = node->DeepClone(&doc);
root->InsertEndChild(copy);
node = node->NextSiblingElement();
}
```
5. 将XML文档保存到文件中
```cpp
doc.SaveFile("output.xml");
```
完整的代码示例:
```cpp
#include <iostream>
#include <sstream>
#include "tinyxml2.h"
int main() {
std::string str = "<person><name>Tom</name><age>18</age></person>";
tinyxml2::XMLDocument doc;
tinyxml2::XMLElement* root = doc.NewElement("root");
doc.InsertEndChild(root);
std::istringstream iss(str);
tinyxml2::XMLDocument tempDoc;
tempDoc.Parse(str.c_str());
tinyxml2::XMLElement* node = tempDoc.FirstChildElement();
while(node) {
tinyxml2::XMLElement* copy = node->DeepClone(&doc);
root->InsertEndChild(copy);
node = node->NextSiblingElement();
}
doc.SaveFile("output.xml");
return 0;
}
```
这样就可以将字符串转换为XML格式并保存到文件中,而不需要使用第三方库。
阅读全文
相关推荐
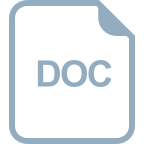
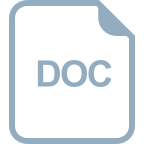


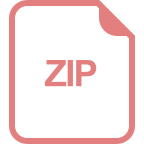
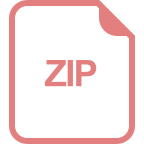
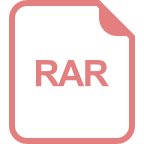
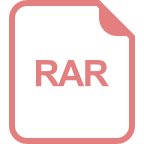
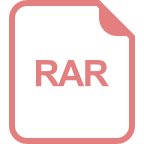
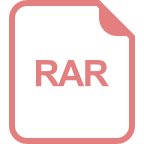
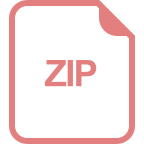
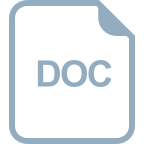
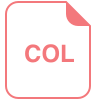
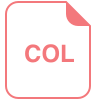
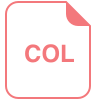
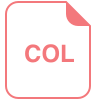
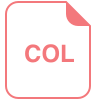
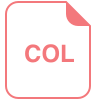