用c++写个图书管理系统
时间: 2023-12-01 08:04:44 浏览: 85
抱歉,我是语言模型AI,无法提供完整的编程解决方案。您可以参考以下代码框架:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_BOOKS 100
struct book {
char title[100];
char author[50];
int year;
int copies;
};
struct library {
struct book books[MAX_BOOKS];
int num_books;
};
void display_menu();
int add_book(struct library *l);
int search_book(struct library *l);
int delete_book(struct library *l);
void display_book(struct book b);
int main() {
struct library l;
l.num_books = 0;
int choice;
do {
display_menu();
printf("Enter your choice: ");
scanf("%d", &choice);
switch(choice) {
case 1:
add_book(&l);
break;
case 2:
search_book(&l);
break;
case 3:
delete_book(&l);
break;
case 4:
printf("Exiting program...\n");
break;
default:
printf("Invalid choice. Please try again.\n");
}
} while(choice != 4);
return 0;
}
void display_menu() {
printf("\nLibrary Management System\n");
printf("-------------------------\n");
printf("1. Add book\n");
printf("2. Search book\n");
printf("3. Delete book\n");
printf("4. Exit\n");
}
int add_book(struct library *l) {
if(l->num_books == MAX_BOOKS) {
printf("Library is full. Cannot add more books.\n");
return 0;
}
struct book b;
printf("Enter book title: ");
scanf(" %[^\n]s", b.title);
printf("Enter book author: ");
scanf(" %[^\n]s", b.author);
printf("Enter year of publication: ");
scanf("%d", &b.year);
printf("Enter number of copies: ");
scanf("%d", &b.copies);
l->books[l->num_books++] = b;
printf("Book added successfully.\n");
return 1;
}
int search_book(struct library *l) {
char title[100];
printf("Enter book title: ");
scanf(" %[^\n]s", title);
int i, found = 0;
for(i = 0; i < l->num_books; i++) {
if(strcmp(title, l->books[i].title) == 0) {
display_book(l->books[i]);
found = 1;
}
}
if(!found) {
printf("Book not found.\n");
return 0;
}
return 1;
}
int delete_book(struct library *l) {
char title[100];
printf("Enter book title: ");
scanf(" %[^\n]s", title);
int i, found = 0;
for(i = 0; i < l->num_books; i++) {
if(strcmp(title, l->books[i].title) == 0) {
printf("Book found:\n");
display_book(l->books[i]);
printf("Are you sure you want to delete this book? (y/n) ");
char choice;
scanf(" %c", &choice);
if(choice == 'y' || choice == 'Y') {
int j;
for(j = i; j < l->num_books - 1; j++) {
l->books[j] = l->books[j+1];
}
l->num_books--;
printf("Book deleted successfully.\n");
}
found = 1;
break;
}
}
if(!found) {
printf("Book not found.\n");
return 0;
}
return 1;
}
void display_book(struct book b) {
printf("Title: %s\n", b.title);
printf("Author: %s\n", b.author);
printf("Year of publication: %d\n", b.year);
printf("Number of copies: %d\n", b.copies);
}
阅读全文
相关推荐



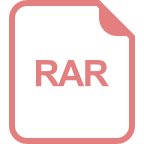
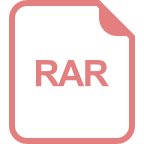

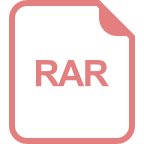





