文章中字符出现概率统计c++fstream
时间: 2024-02-23 09:59:04 浏览: 22
如果你想对一个文件中的字符出现概率进行统计,可以使用C++中的fstream库来读取文件,并且对读取到的字符进行统计。具体实现如下:
```c++
#include <iostream>
#include <fstream>
#include <map>
#include <string>
using namespace std;
int main() {
ifstream fin("filename.txt");
if (!fin.is_open()) {
cout << "Failed to open the file!" << endl;
return 0;
}
map<char, int> mp;
char ch;
while (fin.get(ch)) {
mp[ch]++;
}
fin.close();
for (auto it = mp.begin(); it != mp.end(); it++) {
cout << it->first << ": " << it->second << endl;
}
return 0;
}
```
这段代码中,我们首先使用ifstream库打开文件,然后使用while循环读取文件中的每个字符,并对其进行统计。最后关闭文件,遍历map输出每个字符和其出现的次数。注意,在使用fstream库读取文件时,需要判断文件是否成功打开,否则可能会出现读取失败的情况。
相关问题
文章中字符出现概率统计c++fstream 库
如果你想将字符出现概率统计结果保存到文件中,可以使用C++中的fstream库来写入文件。具体实现如下:
```c++
#include <iostream>
#include <fstream>
#include <map>
#include <string>
using namespace std;
int main() {
ifstream fin("filename.txt");
if (!fin.is_open()) {
cout << "Failed to open the file!" << endl;
return 0;
}
map<char, int> mp;
char ch;
while (fin.get(ch)) {
mp[ch]++;
}
fin.close();
ofstream fout("result.txt");
if (!fout.is_open()) {
cout << "Failed to create the result file!" << endl;
return 0;
}
for (auto it = mp.begin(); it != mp.end(); it++) {
fout << it->first << ": " << it->second << endl;
}
fout.close();
return 0;
}
```
这段代码中,我们首先使用ifstream库打开文件并进行字符出现概率统计,然后使用ofstream库创建一个新的文件,并将统计结果写入其中。需要注意的是,在使用ofstream库写入文件时,需要判断文件是否成功创建,否则可能会出现写入失败的情况。
文章中字符出现概率统计c++
可以使用一个数组来记录每个字符出现的次数,然后再根据总字符数计算出每个字符的出现概率。以下是一个简单的 C++ 代码示例:
```c++
#include <iostream>
#include <fstream>
using namespace std;
int main() {
int count[256] = {0}; // 初始化每个字符出现次数为0
int total = 0; // 总字符数
// 读取文件中的字符
ifstream infile("example.txt");
char c;
while (infile.get(c)) {
count[c]++;
total++;
}
// 输出每个字符出现概率
for (int i = 0; i < 256; i++) {
if (count[i] > 0) {
cout << (char)i << ": " << (double)count[i] / total << endl;
}
}
return 0;
}
```
上述代码中,我们首先定义一个长度为256的数组 `count`,用于记录每个字符出现的次数。然后我们通过读取文件中的字符,将每个字符出现次数加1,并累计总字符数。最后,我们遍历数组 `count`,输出每个字符出现的概率。需要注意的是,字符的 ASCII 码值范围是0~255,因此我们需要定义一个长度为256的数组。
相关推荐
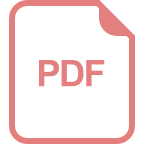












