softmax分类器代码
时间: 2023-07-09 18:52:24 浏览: 54
好的,以下是MATLAB实现MNIST数据集上的softmax回归的示例代码:
```matlab
% Load the MNIST dataset
load mnist.mat
% Reshape the training and testing data into 784x60000 and 784x10000 matrices, respectively
train_data = reshape(train_data, 784, []);
test_data = reshape(test_data, 784, []);
% Normalize the training and testing data to have zero mean and unit variance
[train_data, mu, sigma] = zscore(train_data');
test_data = normalize(test_data', mu, sigma)';
% Convert the training and testing labels to one-hot encoding
num_classes = 10;
train_labels = full(ind2vec(train_labels' + 1, num_classes));
test_labels = full(ind2vec(test_labels' + 1, num_classes));
% Initialize the weights and biases
W = randn(size(train_data, 2), num_classes) * 0.1;
b = zeros(1, num_classes);
% Set the hyperparameters
learning_rate = 0.1;
num_epochs = 50;
batch_size = 100;
% Train the softmax classifier using mini-batch gradient descent
num_batches = size(train_data, 1) / batch_size;
for epoch = 1:num_epochs
for batch = 1:num_batches
% Get the current mini-batch
batch_start = (batch - 1) * batch_size + 1;
batch_end = batch * batch_size;
X = train_data(batch_start:batch_end, :);
Y = train_labels(batch_start:batch_end, :);
% Compute the forward pass
Z = X * W + b;
A = softmax(Z);
% Compute the loss and accuracy
loss = cross_entropy(A, Y);
acc = accuracy(A, Y);
% Compute the gradients using backpropagation
dZ = A - Y;
dW = X' * dZ;
db = sum(dZ, 1);
% Update the weights and biases using mini-batch gradient descent
W = W - learning_rate * dW;
b = b - learning_rate * db;
end
% Evaluate the model on the test set
Z = test_data * W + b;
A = softmax(Z);
loss = cross_entropy(A, test_labels);
acc = accuracy(A, test_labels);
fprintf('Epoch %d, loss = %f, accuracy = %f\n', epoch, loss, acc);
end
```
其中,`softmax`函数用于计算softmax激活函数,`cross_entropy`函数用于计算交叉熵损失,`accuracy`函数用于计算分类准确率。这些函数的实现可以参考以下代码:
```matlab
function A = softmax(Z)
% Compute the softmax activation function
A = exp(Z) ./ sum(exp(Z), 2);
end
function loss = cross_entropy(A, Y)
% Compute the cross-entropy loss
epsilon = 1e-10;
A = max(A, epsilon);
loss = -sum(Y .* log(A), 2);
end
function acc = accuracy(A, Y)
% Compute the classification accuracy
[~, y_pred] = max(A, [], 2);
[~, y_true] = max(Y, [], 2);
acc = mean(y_pred == y_true);
end
```
希望对您有所帮助!
相关推荐
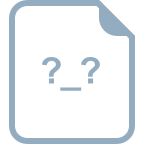
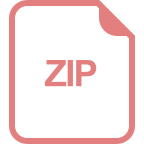
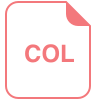
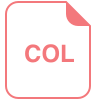












