pyqt播放视频然后截图
时间: 2023-07-01 08:18:51 浏览: 80
可以使用PyQt中的QMediaPlayer和QVideoWidget来播放视频,然后使用QPixmap来截取QVideoWidget中的当前帧作为截图。以下是一个简单的示例代码:
```python
from PyQt5.QtCore import Qt, QUrl
from PyQt5.QtWidgets import QApplication, QWidget, QPushButton, QLabel, QVBoxLayout, QHBoxLayout, QFileDialog, QSizePolicy, QSlider, QStyle, QSizePolicy, QSpacerItem, QVideoWidget
from PyQt5.QtMultimedia import QMediaContent, QMediaPlayer
from PyQt5.QtGui import QPixmap
class VideoPlayer(QWidget):
def __init__(self):
super().__init__()
self.setWindowTitle("Video Player")
# 创建播放器和视频窗口
self.media_player = QMediaPlayer(None, QMediaPlayer.VideoSurface)
self.video_widget = QVideoWidget()
self.media_player.setVideoOutput(self.video_widget)
# 创建截图按钮
self.screenshot_button = QPushButton("Screenshot", self)
self.screenshot_button.clicked.connect(self.take_screenshot)
# 创建标签来显示截图
self.screenshot_label = QLabel()
self.screenshot_label.setAlignment(Qt.AlignCenter)
self.screenshot_label.setSizePolicy(QSizePolicy.Expanding, QSizePolicy.Expanding)
self.screenshot_label.setMinimumSize(240, 135)
# 创建布局
video_layout = QVBoxLayout()
video_layout.addWidget(self.video_widget)
button_layout = QHBoxLayout()
button_layout.addStretch(1)
button_layout.addWidget(self.screenshot_button)
button_layout.addStretch(1)
screenshot_layout = QVBoxLayout()
screenshot_layout.addWidget(QLabel("Screenshot:"))
screenshot_layout.addWidget(self.screenshot_label)
main_layout = QVBoxLayout()
main_layout.addLayout(video_layout)
main_layout.addLayout(button_layout)
main_layout.addLayout(screenshot_layout)
self.setLayout(main_layout)
# 打开视频文件
file_path, _ = QFileDialog.getOpenFileName(self, "Open Video File", "", "Video Files (*.mp4 *.avi *.mkv)")
if file_path:
self.media_player.setMedia(QMediaContent(QUrl.fromLocalFile(file_path)))
self.media_player.play()
def take_screenshot(self):
# 截取当前帧
pixmap = self.video_widget.grab()
self.screenshot_label.setPixmap(pixmap)
if __name__ == '__main__':
app = QApplication([])
player = VideoPlayer()
player.show()
app.exec_()
```
这个程序会打开一个视频文件,可以播放视频,并且有一个截图按钮,点击该按钮可以截取视频当前帧并在界面上显示。
相关推荐
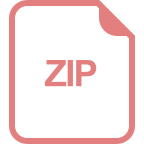
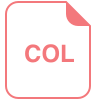













