有100个文件,每个名字都不相同,但是有些文件内容是一样的,用java代码实现判断这一百个文件中相同的文件有哪些,
时间: 2024-05-28 11:10:03 浏览: 66
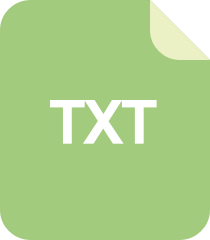
判断文件是否是同一文件

可以使用哈希表来解决这个问题,具体步骤如下:
1. 遍历所有文件,对于每个文件计算其哈希值;
2. 将哈希值作为键,该哈希值对应的文件名作为值,存入哈希表中;
3. 如果哈希表中已经存在该哈希值,则将该文件名添加到该哈希值对应的值中。
以下是Java代码实现:
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class FindDuplicateFiles {
public static void main(String[] args) {
File folder = new File("path/to/folder");
File[] files = folder.listFiles();
Map<String, List<String>> map = new HashMap<>();
MessageDigest md;
try {
md = MessageDigest.getInstance("MD5");
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
return;
}
for (File file : files) {
if (!file.isFile()) {
continue;
}
FileInputStream fis;
try {
fis = new FileInputStream(file);
} catch (FileNotFoundException e) {
e.printStackTrace();
continue;
}
byte[] buffer = new byte[1024];
int numRead;
try {
do {
numRead = fis.read(buffer);
if (numRead > 0) {
md.update(buffer, 0, numRead);
}
} while (numRead != -1);
fis.close();
} catch (IOException e) {
e.printStackTrace();
continue;
}
byte[] digest = md.digest();
StringBuffer hexString = new StringBuffer();
for (int i = 0; i < digest.length; i++) {
String hex = Integer.toHexString(0xff & digest[i]);
if (hex.length() == 1)
hexString.append('0');
hexString.append(hex);
}
String key = hexString.toString();
List<String> fileList = map.get(key);
if (fileList == null) {
fileList = new ArrayList<>();
map.put(key, fileList);
}
fileList.add(file.getName());
}
for (Map.Entry<String, List<String>> entry : map.entrySet()) {
List<String> fileList = entry.getValue();
if (fileList.size() > 1) {
System.out.println("Duplicate files:");
for (String fileName : fileList) {
System.out.println(fileName);
}
}
}
}
}
其中,"path/to/folder"改为文件夹的路径。运行后,程序会输出所有重复的文件。
阅读全文
相关推荐
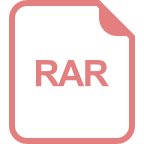
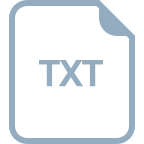
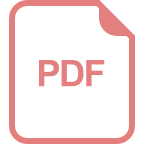
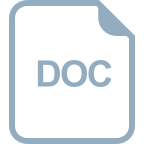
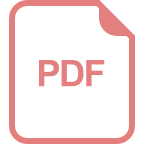
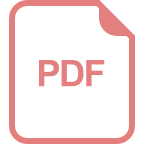
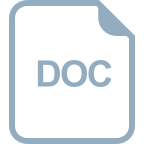
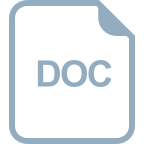
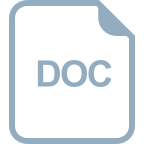
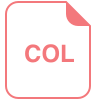
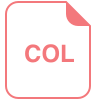
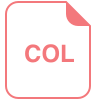