java中创建电脑类,属性有编号,品牌和价格,重写equals方法判断两台电脑价格是否相等
时间: 2024-03-16 22:41:51 浏览: 42
在Java中创建电脑类,可以按照以下方式实现:
```java
public class Computer {
private int id; // 编号
private String brand; // 品牌
private double price; // 价格
// 构造方法
public Computer(int id, String brand, double price) {
this.id = id;
this.brand = brand;
this.price = price;
}
// getter和setter方法
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getBrand() {
return brand;
}
public void setBrand(String brand) {
this.brand = brand;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
// 重写equals方法,判断两台电脑价格是否相等
@Override
public boolean equals(Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof Computer)) {
return false;
}
Computer c = (Computer) obj;
return Double.compare(price, c.price) == 0;
}
}
```
在上述代码中,我们重写了equals方法,通过比较两台电脑的价格是否相等来判断它们是否相等。需要注意的是,equals方法的参数类型为Object,需要进行类型转换。在进行Double类型的比较时,我们使用了Double.compare方法,避免了因为浮点数精度问题导致的比较错误。
相关推荐
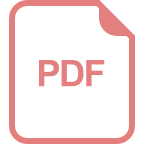














