re.match函数详解
时间: 2024-05-02 21:20:56 浏览: 9
re.match() 函数尝试从字符串的起始位置匹配一个模式,如果匹配成功,就返回一个匹配的对象,否则返回 None。
re.match(pattern, string, flags=0)
参数说明:
- pattern:正则表达式的字符串或原生字符串表示。
- string:待匹配字符串。
- flags:标志位,用于控制正则表达式的匹配方式,如是否区分大小写、多行匹配等。
返回值:
- 匹配成功返回一个匹配对象,否则返回 None。
匹配对象的方法:
- group():返回整个匹配对象(或指定子组)匹配到的字符串。
- start():返回匹配对象(或指定子组)匹配到的字符串的起始位置。
- end():返回匹配对象(或指定子组)匹配到的字符串的结束位置。
- span():返回匹配对象(或指定子组)匹配到的字符串的起始位置和结束位置。
举例:
```python
import re
# 匹配单个字符
result = re.match('h', 'hello')
print(result.group()) # 输出:h
# 匹配多个字符
result = re.match('hel', 'hello')
print(result.group()) # 输出:hel
# 匹配字符集合
result = re.match('[abc]', 'a')
print(result.group()) # 输出:a
# 匹配重复字符
result = re.match('he*', 'hee')
print(result.group()) # 输出:hee
# 匹配任意字符
result = re.match('.', 'h')
print(result.group()) # 输出:h
# 匹配数字
result = re.match('\d+', '123')
print(result.group()) # 输出:123
# 匹配空白字符
result = re.match('\s+', ' ')
print(result.group()) # 输出:2个空格
# 匹配单词字符
result = re.match('\w+', 'hello')
print(result.group()) # 输出:hello
# 匹配反斜杠字符
result = re.match('\\d', '1')
print(result.group()) # 输出:1
# 匹配换行符
result = re.match('\n', '\n')
print(result.group()) # 输出:\n
# 匹配子组
result = re.match('(he)(llo)', 'hello')
print(result.group()) # 输出:hello
print(result.group(1)) # 输出:he
print(result.group(2)) # 输出:llo
# 匹配起始位置
result = re.match('^he', 'hello')
print(result.group()) # 输出:he
# 匹配结束位置
result = re.match('lo$', 'hello')
print(result.group()) # 输出:lo
```
相关推荐
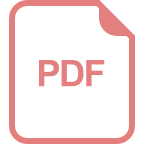
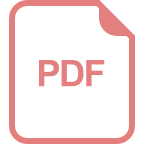
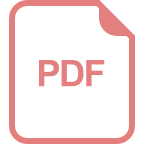













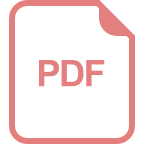
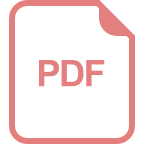