打算用Python 开发一个桌面应用,实现排量获取txt文件,抽取数据并保存抽取记录,可通过查找选择查看历史数据,能说说怎么实现吗?需要用到那些库?
时间: 2024-02-18 20:05:05 浏览: 191
当你想开发一个桌面应用程序时,可以使用 Python 的 GUI 库 Tkinter、PyQt 或 wxPython,这里我推荐使用 PyQt5。在实现排量获取 txt 文件,抽取数据并保存抽取记录时,你可能需要用到以下库:
- PyQt5(GUI 库)
- os(操作系统接口库)
- re(正则表达式库)
- pandas(数据处理库)
以下是一些参考代码:
```python
import sys
import os
import re
import pandas as pd
from PyQt5.QtWidgets import QApplication, QMainWindow, QFileDialog, QMessageBox
class MyWindow(QMainWindow):
def __init__(self):
super().__init__()
# 设置窗口标题和大小
self.setWindowTitle('抽取数据')
self.resize(500, 300)
# 添加菜单栏
menu = self.menuBar().addMenu('文件')
open_action = menu.addAction('打开')
open_action.triggered.connect(self.open_file)
# 添加文本框和按钮
self.textEdit = QTextEdit()
self.setCentralWidget(self.textEdit)
self.button = QPushButton('抽取数据', self)
self.button.move(200, 250)
self.button.clicked.connect(self.extract_data)
# 定义变量
self.file_path = ''
self.data = None
def open_file(self):
# 弹出文件选择对话框
file_path, _ = QFileDialog.getOpenFileName(self, '选择文件', '', 'Text Files (*.txt)')
if file_path:
self.file_path = file_path
with open(file_path, 'r') as f:
self.textEdit.setText(f.read())
def extract_data(self):
# 获取文本框中的内容
text = self.textEdit.toPlainText()
# 使用正则表达式抽取数据
pattern = r'排量:(\d+\.\d+)L'
results = re.findall(pattern, text)
# 将抽取结果存储到 pandas DataFrame 中
data = pd.DataFrame({'排量': results})
# 保存抽取记录到文件中
if self.file_path:
record_path = os.path.splitext(self.file_path)[0] + '_record.csv'
data.to_csv(record_path, index=False)
QMessageBox.information(self, '提示', '保存成功!')
else:
QMessageBox.warning(self, '警告', '请先打开文件!')
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MyWindow()
window.show()
sys.exit(app.exec_())
```
上面的代码实现了一个简单的 GUI 程序,用于抽取文本文件中的排量数据,并将抽取记录保存到 CSV 文件中。你可以根据需要进行修改和完善。
阅读全文
相关推荐
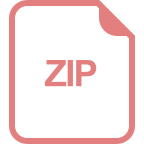
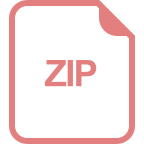
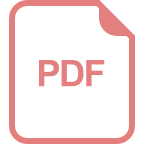
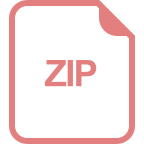
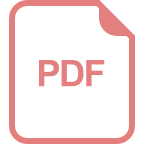
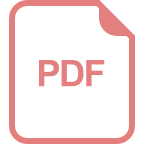
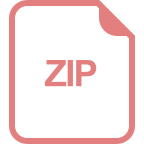
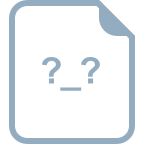
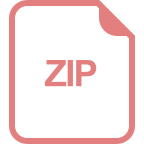
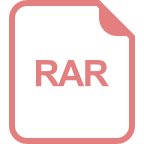
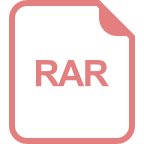
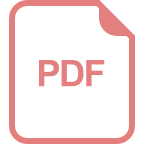
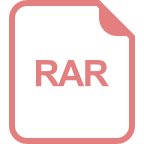
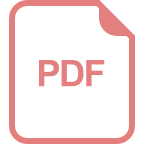
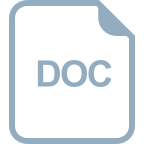