java 分布式锁代码
时间: 2023-07-06 19:43:09 浏览: 54
以下是一个基于 Redis 的分布式锁 Java 代码示例:
```java
import redis.clients.jedis.Jedis;
public class DistributedLock {
private Jedis jedis;
private String lockKey;
private int expireTime = 30000; // 锁过期时间,默认30秒
private boolean locked = false;
public DistributedLock(Jedis jedis, String lockKey) {
this.jedis = jedis;
this.lockKey = lockKey;
}
/**
* 尝试获取分布式锁
* @return 是否获取成功
*/
public boolean tryLock() {
long expires = System.currentTimeMillis() + expireTime + 1;
String expiresStr = String.valueOf(expires); // 锁到期时间
if (jedis.setnx(lockKey, expiresStr) == 1) {
// 获取锁成功
locked = true;
return true;
}
String currentValueStr = jedis.get(lockKey); // 获取当前锁的到期时间
if (currentValueStr != null && Long.parseLong(currentValueStr) < System.currentTimeMillis()) {
// 锁已过期,尝试获取锁
String oldValueStr = jedis.getSet(lockKey, expiresStr);
if (oldValueStr != null && oldValueStr.equals(currentValueStr)) {
// 获取锁成功
locked = true;
return true;
}
}
// 获取锁失败
return false;
}
/**
* 释放分布式锁
*/
public void unlock() {
if (locked) {
jedis.del(lockKey);
locked = false;
}
}
}
```
使用方法如下:
```java
Jedis jedis = new Jedis("localhost", 6379);
String lockKey = "my_lock";
DistributedLock lock = new DistributedLock(jedis, lockKey);
if (lock.tryLock()) {
try {
// 获取锁成功,执行业务代码
} finally {
lock.unlock(); // 释放锁
}
} else {
// 获取锁失败,处理异常情况
}
```
需要注意的是,在使用分布式锁时要保证锁的过期时间足够长,同时也要注意解锁的时机。另外,可以考虑加入重试机制来避免因为网络等原因导致的获取锁失败。
相关推荐
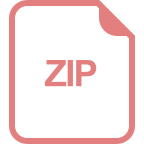
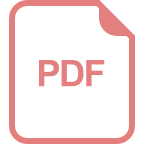













