用c++写一个使用顺序表的员工管理系统
时间: 2024-05-15 20:12:51 浏览: 97
好的,以下是一个简单的使用顺序表实现员工管理系统的C++代码:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
const int MAX_SIZE = 100; // 最大员工数量
// 员工结构体
struct Employee {
int id; // 员工编号
char name[20]; // 员工姓名
int age; // 员工年龄
char gender; // 员工性别
};
// 员工管理系统类
class EmployeeManagementSystem {
public:
EmployeeManagementSystem() : size(0) {} // 构造函数,初始员工数量为0
~EmployeeManagementSystem() {} // 析构函数
void addEmployee(); // 添加员工
void deleteEmployee(); // 删除员工
void modifyEmployee(); // 修改员工信息
void searchEmployee(); // 查找员工信息
void displayAllEmployees(); // 显示所有员工信息
private:
Employee employees[MAX_SIZE]; // 员工数组
int size; // 员工数量
};
void EmployeeManagementSystem::addEmployee() {
if (size >= MAX_SIZE) {
cout << "员工数量已达到上限,无法添加新员工!" << endl;
return;
}
Employee e;
cout << "请输入员工编号:";
cin >> e.id;
cout << "请输入员工姓名:";
cin >> e.name;
cout << "请输入员工年龄:";
cin >> e.age;
cout << "请输入员工性别(M/F):";
cin >> e.gender;
employees[size++] = e; // 将新员工添加到数组末尾
cout << "添加成功!" << endl;
}
void EmployeeManagementSystem::deleteEmployee() {
if (size == 0) {
cout << "员工列表为空,无法删除员工!" << endl;
return;
}
int id;
cout << "请输入要删除的员工编号:";
cin >> id;
int index = -1;
for (int i = 0; i < size; i++) {
if (employees[i].id == id) {
index = i;
break;
}
}
if (index == -1) {
cout << "未找到要删除的员工!" << endl;
return;
}
for (int i = index; i < size - 1; i++) {
employees[i] = employees[i + 1]; // 将后面的员工向前移动
}
size--; // 员工数量减1
cout << "删除成功!" << endl;
}
void EmployeeManagementSystem::modifyEmployee() {
if (size == 0) {
cout << "员工列表为空,无法修改员工信息!" << endl;
return;
}
int id;
cout << "请输入要修改的员工编号:";
cin >> id;
int index = -1;
for (int i = 0; i < size; i++) {
if (employees[i].id == id) {
index = i;
break;
}
}
if (index == -1) {
cout << "未找到要修改的员工!" << endl;
return;
}
Employee e;
cout << "请输入员工姓名(原值为" << employees[index].name << "):";
cin >> e.name;
cout << "请输入员工年龄(原值为" << employees[index].age << "):";
cin >> e.age;
cout << "请输入员工性别(M/F,原值为" << employees[index].gender << "):";
cin >> e.gender;
e.id = id;
employees[index] = e; // 将修改后的员工信息替换原有信息
cout << "修改成功!" << endl;
}
void EmployeeManagementSystem::searchEmployee() {
if (size == 0) {
cout << "员工列表为空,无法查找员工信息!" << endl;
return;
}
int id;
cout << "请输入要查找的员工编号:";
cin >> id;
int index = -1;
for (int i = 0; i < size; i++) {
if (employees[i].id == id) {
index = i;
break;
}
}
if (index == -1) {
cout << "未找到要查找的员工!" << endl;
return;
}
Employee e = employees[index];
cout << "员工编号:" << e.id << endl;
cout << "员工姓名:" << e.name << endl;
cout << "员工年龄:" << e.age << endl;
cout << "员工性别:" << e.gender << endl;
}
void EmployeeManagementSystem::displayAllEmployees() {
if (size == 0) {
cout << "员工列表为空!" << endl;
return;
}
cout << "员工列表:" << endl;
for (int i = 0; i < size; i++) {
Employee e = employees[i];
cout << "员工编号:" << e.id << endl;
cout << "员工姓名:" << e.name << endl;
cout << "员工年龄:" << e.age << endl;
cout << "员工性别:" << e.gender << endl;
}
}
int main() {
EmployeeManagementSystem ems;
while (true) {
cout << "请选择操作:" << endl;
cout << "1. 添加员工" << endl;
cout << "2. 删除员工" << endl;
cout << "3. 修改员工信息" << endl;
cout << "4. 查找员工信息" << endl;
cout << "5. 显示所有员工信息" << endl;
cout << "6. 退出" << endl;
int choice;
cin >> choice;
switch (choice) {
case 1:
ems.addEmployee();
break;
case 2:
ems.deleteEmployee();
break;
case 3:
ems.modifyEmployee();
break;
case 4:
ems.searchEmployee();
break;
case 5:
ems.displayAllEmployees();
break;
case 6:
return 0;
default:
cout << "无效操作!" << endl;
}
cout << endl;
}
return 0;
}
```
这个员工管理系统使用顺序表来存储员工信息,可以添加、删除、修改、查找和显示所有员工信息。运行时,会显示一个菜单供用户选择操作,直到用户选择退出为止。
阅读全文
相关推荐



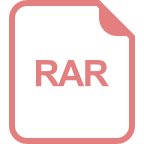





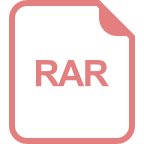





