PyQt实现运行其他python文件
时间: 2023-12-09 08:04:02 浏览: 165
要在PyQt中运行其他Python文件,你可以使用Python的`subprocess`模块。具体步骤如下:
1. 在你的PyQt窗口中,导入`subprocess`模块,如:
```python
import subprocess
```
2. 在事件处理程序中,调用`subprocess`模块的`run()`函数,如:
```python
def run_other_py(self):
# 运行名为"other.py"的Python文件
subprocess.run(['python', 'other.py'])
```
在这个例子中,`subprocess.run()`函数将调用系统中的Python解释器,并传递"other.py"作为参数来运行这个Python文件。你可以根据需要更改参数。
需要注意的是,使用`subprocess.run()`函数会阻塞当前进程,直到被调用的Python文件运行完毕。如果你希望在后台运行这个Python文件并立即返回,可以使用`subprocess.Popen()`函数。如:
```python
def run_other_py(self):
# 在后台运行名为"other.py"的Python文件
subprocess.Popen(['python', 'other.py'])
```
这样,被调用的Python文件将在后台运行,并且`subprocess.Popen()`函数将立即返回。
相关问题
PyQt实现button点击触发其他python文件的运行
可以在button的点击事件中调用其他Python文件的函数或方法来实现。具体步骤如下:
1. 导入需要调用的Python文件的模块或函数。
2. 在button的点击事件中调用需要运行的函数或方法。
下面是一个简单的示例代码:
```
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QPushButton
from other_module import run_function # 导入需要调用的Python文件的函数
class Example(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
btn = QPushButton('Run Function', self)
btn.clicked.connect(self.runOtherFile) # 按钮点击事件连接到runOtherFile方法
self.setGeometry(300, 300, 300, 200)
self.setWindowTitle('Example')
self.show()
def runOtherFile(self):
run_function() # 调用其他Python文件中的函数
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = Example()
sys.exit(app.exec_())
```
在上面的示例中,我们导入了一个名为`run_function`的函数,然后在button的点击事件`runOtherFile`方法中调用该函数。当我们点击按钮时,就会执行`run_function`函数。
PyQt实现button点击触发python文件中其他.py模块的运行
你可以使用PyQt中的QPushButton模块来实现这个功能。具体步骤如下:
1. 在你的PyQt窗口中,创建一个QPushButton对象。
2. 给这个QPushButton对象绑定一个clicked信号,以便在按钮被点击时触发一个事件。
3. 在事件处理程序中,调用你想要运行的.py文件,可以使用Python的subprocess模块来实现。
下面是一个简单的例子:
```python
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton
import subprocess
class MyWindow(QMainWindow):
def __init__(self):
super().__init__()
# 创建一个按钮
self.button = QPushButton('点击运行其他.py文件', self)
self.button.setGeometry(50, 50, 200, 50)
# 绑定按钮的clicked信号到事件处理程序
self.button.clicked.connect(self.run_other_py)
def run_other_py(self):
# 调用其他.py文件
subprocess.call(['python', 'other.py'])
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MyWindow()
window.show()
sys.exit(app.exec_())
```
当你点击按钮时,它将调用名为"other.py"的文件。请注意,在这个例子中,我使用了Python的subprocess模块来调用其他.py文件。
阅读全文
相关推荐
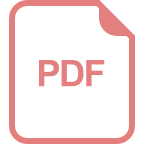
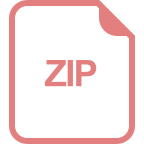

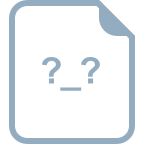
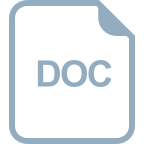




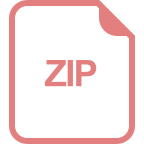
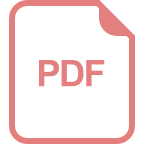
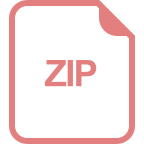
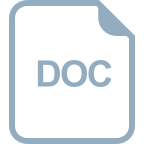
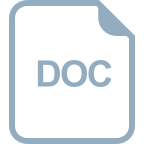
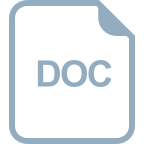
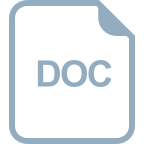