基于vue写一个消除方块的游戏代码
时间: 2024-05-05 12:21:30 浏览: 10
好的,下面是一个简单的消除方块游戏的代码示例,采用Vue框架实现。
HTML部分:
```html
<div id="app">
<div class="game-container">
<div class="game-board">
<div class="row" v-for="(row, rowIndex) in board" :key="rowIndex">
<div class="cell" v-for="(cell, cellIndex) in row" :key="cellIndex"
:class="{ 'selected': cell.selected }"
@click="cellClicked(rowIndex, cellIndex)">
{{ cell.color }}
</div>
</div>
</div>
<div class="game-controls">
<button @click="shuffleBoard">Shuffle</button>
<button @click="clearBoard">Clear</button>
<button @click="checkBoard">Check</button>
</div>
</div>
</div>
```
CSS部分:
```css
.game-container {
display: flex;
flex-direction: column;
align-items: center;
margin-top: 50px;
}
.game-board {
display: flex;
flex-direction: column;
align-items: center;
border: 2px solid black;
padding: 10px;
}
.row {
display: flex;
}
.cell {
display: flex;
justify-content: center;
align-items: center;
width: 50px;
height: 50px;
border: 1px solid black;
margin: 5px;
cursor: pointer;
}
.cell.selected {
background-color: yellow;
}
.game-controls {
margin-top: 20px;
}
button {
font-size: 16px;
margin-right: 10px;
}
```
JavaScript部分:
```js
const colors = ['red', 'green', 'blue', 'yellow', 'purple'];
const app = new Vue({
el: '#app',
data: {
board: [],
selectedColor: '',
numMoves: 0
},
mounted() {
this.resetBoard();
},
methods: {
resetBoard() {
this.numMoves = 0;
this.board = Array.from({ length: 8 }, () =>
Array.from({ length: 8 }, () => ({
color: colors[Math.floor(Math.random() * colors.length)],
selected: false
}))
);
},
shuffleBoard() {
this.numMoves++;
this.board.forEach(row =>
row.forEach(cell => {
cell.color = colors[Math.floor(Math.random() * colors.length)];
cell.selected = false;
})
);
},
clearBoard() {
this.numMoves++;
this.board.forEach(row =>
row.forEach(cell => {
cell.color = '';
cell.selected = false;
})
);
},
checkBoard() {
this.numMoves++;
let groups = [];
// Check horizontal groups
for (let row = 0; row < this.board.length; row++) {
let groupStart = 0;
for (let col = 1; col <= this.board[row].length; col++) {
if (
col === this.board[row].length ||
this.board[row][col].color !== this.board[row][groupStart].color
) {
if (col - groupStart >= 3) {
groups.push({
direction: 'horizontal',
startRow: row,
startCol: groupStart,
endRow: row,
endCol: col - 1
});
}
groupStart = col;
}
}
}
// Check vertical groups
for (let col = 0; col < this.board[0].length; col++) {
let groupStart = 0;
for (let row = 1; row <= this.board.length; row++) {
if (
row === this.board.length ||
this.board[row][col].color !== this.board[groupStart][col].color
) {
if (row - groupStart >= 3) {
groups.push({
direction: 'vertical',
startRow: groupStart,
startCol: col,
endRow: row - 1,
endCol: col
});
}
groupStart = row;
}
}
}
if (groups.length > 0) {
groups.forEach(group => {
for (let row = group.startRow; row <= group.endRow; row++) {
for (let col = group.startCol; col <= group.endCol; col++) {
this.board[row][col].selected = true;
}
}
});
} else {
alert('No groups found!');
}
},
cellClicked(row, col) {
const cell = this.board[row][col];
if (cell.color === '') {
return;
}
if (this.selectedColor === '') {
this.selectedColor = cell.color;
cell.selected = true;
} else if (this.selectedColor === cell.color) {
cell.selected = true;
this.selectedColor = '';
} else {
this.board.forEach(row =>
row.forEach(cell => {
cell.selected = false;
})
);
cell.selected = true;
this.selectedColor = cell.color;
}
}
}
});
```
这个游戏的实现可以随机生成一个8x8的方块棋盘,方块的颜色从给定的颜色数组中随机选择。玩家可以点击任意方块,选择它或取消选择它。如果玩家选择了两个同色方块,那么这两个方块将被消除。当所有同色方块都被消除时,游戏结束。
在游戏中,玩家可以使用Shuffle按钮来重新洗牌,使用Clear按钮来清除所有方块,使用Check按钮来检查是否有三个或更多同色方块相连。如果有,这些方块将被高亮显示,玩家可以选择它们以消除它们。
相关推荐
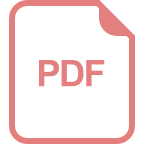
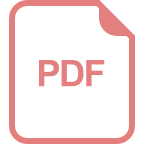
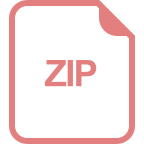















