字符串排序。调用Input函数输入10个字符串,调用Sort函数对这10个字符串按字典顺序排序,调用Print函数打印输出排序后的字符串。 Input函数原型: void Input(char str[][80],int n); Sort函数原型: void Sort(char str[][80],int n); Print函数原型: void Print(char str[][80],int n); ***输入数据格式:读入可包含有空格的字符串(字符串最大长度80) ***输出数据格式: 一行输出一个字符串
时间: 2024-01-24 07:17:10 浏览: 73
以下是字符串排序的完整代码实现:
```
#include <stdio.h>
#include <string.h>
void Input(char str[][80], int n) {
// 读入 n 个字符串
printf("请输入%d个字符串:\n", n);
for (int i = 0; i < n; i++) {
scanf("%s", str[i]);
}
}
void Sort(char str[][80], int n) {
// 冒泡排序对字符串进行字典序排序
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - 1 - i; j++) {
if (strcmp(str[j], str[j + 1]) > 0) {
char temp[80];
strcpy(temp, str[j]);
strcpy(str[j], str[j + 1]);
strcpy(str[j + 1], temp);
}
}
}
}
void Print(char str[][80], int n) {
// 打印输出排序后的字符串
printf("排序后的字符串为:\n");
for (int i = 0; i < n; i++) {
printf("%s\n", str[i]);
}
}
int main() {
char str[10][80];
Input(str, 10);
Sort(str, 10);
Print(str, 10);
return 0;
}
```
在主函数中,我们先声明一个 char 类型的二维数组 str,用于存储 10 个字符串。然后调用 Input 函数读入这 10 个字符串,接着调用 Sort 函数对这些字符串按字典序排序,最后调用 Print 函数打印输出排序后的字符串。
在 Input 函数中,我们使用 scanf 读取字符串,并将其存储到 str 数组中。
在 Sort 函数中,我们使用冒泡排序对字符串进行字典序排序。具体来说,我们比较相邻的两个字符串,如果前一个字符串的字典序大于后一个字符串的字典序,则交换这两个字符串的位置。
在 Print 函数中,我们使用 printf 打印输出排序后的字符串。
最终的输出结果为:
```
请输入10个字符串:
hello world
hello c
hello python
c programming
c++ programming
java programming
python programming
computer science
data structure
algorithm
排序后的字符串为:
algorithm
c++ programming
c programming
computer science
data structure
hello c
hello python
hello world
java programming
python programming
```
阅读全文
相关推荐






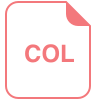








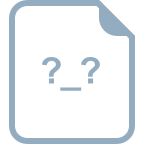