flutter mqtt example
时间: 2023-07-06 14:22:21 浏览: 54
这里是一个简单的Flutter MQTT例子,它连接到一个公共MQTT代理,并订阅一个主题。当收到来自该主题的消息时,它会在UI上显示它们。
首先,您需要在`pubspec.yaml`文件中添加`mqtt_client`依赖项,然后运行`flutter packages get`以安装它。
```
dependencies:
mqtt_client: ^4.0.0
```
然后,您可以使用以下代码创建Flutter应用程序。
```
import 'package:flutter/material.dart';
import 'package:mqtt_client/mqtt_client.dart';
import 'package:mqtt_client/mqtt_server_client.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
MqttServerClient client;
String message = '';
@override
void initState() {
super.initState();
client = MqttServerClient('broker.hivemq.com', '');
client.onConnected = onConnected;
client.onSubscribed = onSubscribed;
client.onDisconnected = onDisconnected;
client.onUnsubscribed = onUnsubscribed;
client.pongCallback = pong;
connect();
}
void connect() async {
try {
await client.connect();
} catch (e) {
print('Exception: $e');
}
}
void subscribe() {
client.subscribe('topic/test', MqttQos.atLeastOnce);
}
void onConnected() {
print('Connected');
subscribe();
}
void onSubscribed(String topic) {
print('Subscribed to $topic');
}
void onDisconnected() {
print('Disconnected');
}
void onUnsubscribed(String topic) {
print('Unsubscribed from $topic');
}
void pong() {
print('Ping response client callback invoked');
}
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('MQTT Chat'),
),
body: Center(
child: Text(message),
),
),
);
}
}
```
在上面的代码中,我们首先创建了一个`MqttServerClient`对象,它连接到公共MQTT代理`broker.hivemq.com`。我们还设置了一些回调函数,以便在连接,订阅和断开连接时得到通知。
在`initState`方法中,我们连接到MQTT服务器。一旦连接成功,我们将订阅`topic/test`主题。
当我们收到来自该主题的消息时,我们将在UI上显示它们。为此,我们可以在`onSubscribed`回调函数中添加以下代码。
```
void onSubscribed(String topic) {
print('Subscribed to $topic');
client.updates.listen((List<MqttReceivedMessage<MqttMessage>> messages) {
final recMess = messages[0].payload as MqttPublishMessage;
final pt =
MqttPublishPayload.bytesToStringAsString(recMess.payload.message);
setState(() {
message = pt;
});
});
}
```
上面的代码将使用`client.updates`流监听来自MQTT服务器的消息。当收到消息时,它将在UI上显示它们。
这是一个简单的Flutter MQTT例子。您可以根据自己的需要进行修改和扩展。
相关推荐
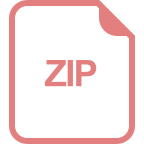














